Branching
Assembler coding - Lesson 3
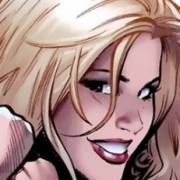
Here we will discuss more about branching to other places in your program.
It would be handy to be able to jump to different places in a program depending upon conditions that have occurred. For example we could do a check to see if a sprite has reached a certain position on the screen, the program then decides if it has or not, and then acts on the decision.
So how do they work. Well, the C64 contains a status register which holds certain flag data, these are as follows:
- Negative (N)
- Zero (Z)
- Carry (C)
- Interrupt (I)
- Decimal (D)
- Overflow (V)
The conditions of these flags determine the operation of your program.
Each of the flags can have 2 states. They can either be a '1' or a '0' - When a flag is '1' it is termed SET, and '0' NOT SET.
Right, lets assume one section of our code is this:
100 LDA $5000
110 BNE BRANCH_HERE
120 ....
Ok, we get the data stored in location 5000 Hex, and it is placed in the accumulator. If the value fetched is ZERO, then the 'Z' flag is set to a '1'. If the value is not ZERO, then the 'Z' flag is '0'. (The flag values are changed by the processor - we will learn later how to alter them manually).
The BNE instruction (Branch Not Equal) does just that, it branches if the result is not zero. It is able to do this by checking the status flag 'Z'. In this case, if the 'Z' flag = 0, then the program branches to the address specified, otherwise line 120 is executed.
If we did this way instead:
100 LDA $5000
110 BEQ BRANCH_HERE
120 ...
Line 100 is the same, fetches the value from address 5000 Hex and places it in accumulator.
The BEQ instruction on line 110, (Branch EQual zero), branches if the value = 0. i.e. this instruction is the opposite to the previous and therefore branches when the 'Z' flag = 1.
If 'Z' = 0 here, line 120 is executed.
These 2 instructions, BNE and BEQ are probably the main branch instructions used, however the remaining branch instructions are also used depending on how you write your code and what you want to do. They basically work the same, but use different flags, i.e. the CARRY flag, OVERFLOW flag and NEGATIVE flag. Have a look at the programmers reference guide.
One important point for the branch instruction. They are all limited to:
- +129 forward memory locations
- -126 backward memory locations
This means the branch range is limited. Of course you can work around this easily.
Ok, but say you don't want to check for ZERO all the time, you want to check if a sprite has reached position 200 in the x direction for example. Well its simple, you can use COMPARE instructions.
These work by firstly placing something in the accumulator, a value to check against. You then use your compare instruction with another value, the processor then calculates the following sum:
ACCUMULATOR - COMPARE VALUE
The result is placed in the STATUS FLAGS, and hence you can branch on the result just like shown earlier. An example is shown below:
10 LDA 53248
20 CMP #200
30 BEQ SPRITE_AT_200
40 ...
Firstly we load the accumulator with the decimal address of Sprite 0 x position. (also LDA $D000)
We then use a compare instruction, to compare with the value 200 decimal.
Lets assume the x position value is 120. The compare instruction calculates 120 - 200.
The result is not ZERO, and therefore the branch instruction is NOT executed. You should be able to see though which flag will be SET.
Now if the x position is 200, then 200 - 200 = 0, and so the branch instruction IS executed.
We also know that the C64 has 3 registers, these are:
- Accumulator
- X register
- Y register
And you can use all three with the branch and compare instructions.
10 LDY 53248
20 CPY #200
30 BEQ SPRITE_AT_200
40 ...
Or, using the X register:
10 LDX 53248
20 CPX #200
30 BEQ SPRITE_AT_200
40 ...
And believe me, you will use them alot.