Using Sprites
Assembler coding - Lesson 5
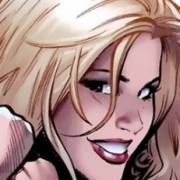
Firstly, you may wish to download 3 in 1 SPRITE EDITOR. A real cool editor package where you can create your own multi coloured sprites or fonts. The download file also contains other programs you may like. To run 3 in 1 editor type SYS 50000 after you load it.
Ok, lets now place a sprite on the screen and then get it moving. Here's roughly what we need to do:
- Clear Screen
- Set up Sprite pointer
- Set up Sprite Colours & Mode
- Set up X & Y position of Sprite
- Move it Down
- Move it Up
So lets write the code:
10 LDA #147
20 JSR $FFD2
30 LDA #00
40 STA 53280
50 STA 53281
60 LDA #255
70 STA 53269
80 STA 53276
90 LDA #02
100 STA 53285
110 LDA #03
120 STA 53286
130 LDX #00
140 COLOUR LDA #04
150 STA 53287,X
160 INX
170 CPX #08
180 BNE COLOUR
190 LDX #64
200 STX 2040
210 INX
220 STX 2041
230 INX
240 STX 2042
250 INX
260 STX 2043
270 INX
280 STX 2044
290 INX
300 STX 2045
310 INX
320 STX 2046
330 INX
340 STX 2047
350 LDY # 70
360 STY 53249
370 STY 53251
380 STY 53253
390 STY 53255
400 STY 53257
410 STY 53259
420 STY 53261
430 STY 53263
440 LDX #30
450 STX 53248
460 LDX #60
470 STX 53250
480 LDX #90
490 STX 53252
500 LDX #120
510 STX 53254
520 LDX #150
530 STX 53256
540 LDX #180
550 STX 52358
560 LDX #210
570 STX 53260
580 LDX #240
590 STX 53262
610 DOWN LDX #100
620 LOOP1 LDY #100
630 LOOP DEY
640 BNE LOOP
650 DEX
660 BNE LOOP1
670 INC 53249
680 INC 53251
690 INC 53253
700 INC 53255
710 INC 53257
720 INC 53259
730 INC 53261
740 INC 53263
750 LDA 53249
760 CMP #200
770 BNE DOWN
780 UP LDX #100
790 LOOP3 LDY #100
800 LOOP2 DEY
810 BNE LOOP2
820 DEX
830 BNE LOOP3
840 DEC 53249
850 DEC 53251
860 DEC 53253
870 DEC 53255
880 DEC 53257
890 DEC 53259
900 DEC 53261
910 DEC 53263
920 LDA 53249
930 CMP #70
940 BNE UP
950 JMP DOWN
So how does it work ?
10 & 20 clears the screen
30,40,50 give the border & background the colour black
60,70,80 turn on all 8 sprites and enable multi coloured sprites
90, 100 sets sprite multicolour register 0 to RED
110,120 sets sprite multicolour register 1 to CYAN
130 - 180 sets each sprite colour to PURPLE using a Compare & Branch Loop
190 - 340 sets each sprite data pointer. The sprites here would be in bank 0 and the value 64 gives them a location in memory of 1000 Hex or 4096 decimal. This is where you would load your sprite data. Of course you would change the colours above to suit your sprites. The formula to calculate where your sprite data is, is shown here:
LOCATION = (BANK * 16384) + (SPRITE POINTER VALUE * 64)
So for our case we have:
LOCATION = ( 0 * 16384) + (64 * 64)
Hence
LOCATION = 4096 Decimal (1000 Hex)
So the first sprite uses 64 as its value, and the next 65 etc until all sprites have there data pointer location.
350 - 430 These set the Y position on the screen for each sprite to 70
440 - 590 These set the X position on the screen for each sprite, starting at 30 for sprite 0, to 240 for sprite 7
600 (Oops I made a mistake and missed this line, no matter)
610 - 770 These lines of code move the sprites down the screen. The code uses a time delay loop to slow the process down, an example of this loop is shown below:
LDX #100
LOOP1 LDY #100
LOOP DEY
BNE LOOP
DEX
BNE LOOP1
This is really simple to follow, Firstly it sets the X and Y registers to 100, then -1 from Y until Y = 0, then -1 from the X register and see if X = 0, if not then branch back to line LDY #100 and start again. The effect is a delay. Without this delay the sprite movement would be too quick to see. You could change these values to suit yourself. Of course the maximum value you can have in each loop is 255.
The way the sprite is moved is simply by incrementing the Y position for each sprite by +1. (You increment to move down as that is the way the screen is setup, i.e. the top left is position (0,0)
The code then checks the position of sprite 0, if this position is 200 then the program falls through the branch to move the sprite up the screen. If the position is 200 then the program branches back to continue to move the sprite down the screen.
780 - 950 Exactly the same as moving down except we move the sprites up by incrementing each sprite Y position and we check the position for 70. If not 70 move the sprite up some more, if position = 70 then this time the program jumps back to line labelled DOWN to again move the sprite down again.
And so the process would go on & on moving the sprite up & down the screen.
Well i hope you could follow that. It is really easy, honest. It looks long but most of the code is for actually setting up the sprite colours and pointers and initial screen positions.
This example doesn't move the sprites in the X direction, but you do that the same. The hardest part with that is getting the sprites in and out of the MSB i.e. past 255 in the X direction. Yes you heard right, the screen in the X direction is split into two visible sections. These are 24 to 255 & then in the next section 0 to 91. We will do that in another section.
In the next lesson we will produce more efficient coding of the same thing, but this time I'll show you how to make those sprites bounce instead of just moving up & down.