Raster Colour Bars
Assembler coding - Lesson 10
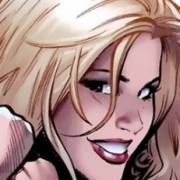
In this lesson we will learn how to code some colourful raster bars that move up the screen.
With this example we are going to do it in the bottom border and we are going to have 4 different coloured bars.
Firstly we need to setup the interrupts. Then we get the colour data from a look up table (which holds the colours for BAR1) and make the border equal to this colour. We then do a short delay to keep on that border colour for a while.
We then get the next colour data from this table and repeat the process until BAR1 is done.
The above is then repeated for the other 3 bars, but these have there own look up tables which holds there own colour data.
If we now left this as it was, all we would have is a static display of colours on the screen, as each time the interrupt occurs the same colours are fetched and placed at the same location on the screen.
To remedy this all we have to do is ROTATE the colours in memory.
EXAMPLE
Just the original look up table for BAR1 was this:
2,10,7,1,1,7,10,2,0,0,0,0
After rotating it in memory the data now looks like this:
10,7,1,1,7,10,2,0,0,0,0,2
So we write a routine to do this for each colour bar and then when the process is now repeated the colours have moved slightly.
Then again we rotate the colours one place, display them and so on. The effect is that the bars scroll up the screen.
Lets view the code.
10 ORG 8192
20 SEI
30 LDA #147
40 JSR $FFD2
50 LDA #$7F
60 STA $DC0D
70 LDA #$FA
80 STA $D012
90 LDA ^IRQ (YOU USE THE UP ARROW)
100 STA $0315
110 LDA #IRQ
120 STA $0314
130 LDA #$F1
140 STA $D01A
150 CLI
160 EVER JMP EVER
170 IRQ LDA #01
180 STA $D019
190 LDA $D011
200 AND #$7F
210 STA $D011
220 LDA #$FA
230 STA $D012
240 LDX #00
250 BACK LDA COLS,X
260 STA 53280
270 JSR DELAY
280 INX
290 CPX #12
300 BNE BACK
310 LDX #00
320 BACK1 LDA COLS1,X
330 STA 53280
340 JSR DELAY
350 INX
360 CPX #12
370 BNE BACK1
380 LDX #00
390 BACK2 LDA COLS2,X
400 STA 53280
410 JSR DELAY
420 INX
430 CPX #12
440 BNE BACK2
450 LDX #00
460 BACK3 LDA COLS3,X
470 STA 53280
480 JSR DELAY
490 INX
500 CPX #11
510 BNE BACK3
520 LDY #02
530 DEL NOP
540 DEY
550 BNE DEL
560 LDX #00
570 STX 53280
580 STX 53281
590 LDY COLS
600 LOOP LDA COLS+1,X
610 STA COLS,X
620 INX
630 CPX #12
640 BNE LOOP
650 TYA
660 STA COLS,X
670 LDX #00
680 LDY COLS1
690 LOOP1 LDA COLS1+1,X
700 STA COLS1,X
710 INX
720 CPX #12
730 BNE LOOP1
740 TYA
750 STA COLS1,X
760 LDX #00
770 LDY COLS2
780 LOOP2 LDA COLS2+1,X
790 STA COLS2,X
800 INX
810 CPX #12
820 BNE LOOP2
830 TYA
840 STA COLS2,X
850 LDX #00
860 LDY COLS3
870 LDA COLS3+1,X
880 STA COLS3,X
890 INX
900 CPX #11
910 BNE LOOP3
920 TYA
930 STA COLS3,X
940 JMP $EA31
950 DELAY LDY #05
960 DEL1 NOP
970 DEY
980 BNE DEL1
990 RTS
1000 COLS DFB 9,8,7,1,1,7,8,9,0,0,0,0
1010 COLS1 DFB 2,10,7,1,1,7,10,2,0,0,0,0
1020 COLS2 DFB 6,14,7,1,1,7,14,6,0,0,0,0
1030 COLS3 DFB 5,13,7,1,1,7,13,5,0,0,0
And the explanation:
THIS IS THE SETUP
10 ORG 8192
20 SEI
30 LDA #147
40 JSR $FFD2
50 LDA #$7F
60 STA $DC0D
70 LDA #$FA
80 STA $D012
90 LDA ^IRQ (YOU USE THE UP ARROW)
100 STA $0315
110 LDA #IRQ
120 STA $0314
130 LDA #$F1
140 STA $D01A
150 CLI
160 EVER JMP EVER
THE REST IS THE INTERRUPT ROUTINE (ALSO BROKEN DOWN)
170 IRQ LDA #01
180 STA $D019
190 LDA $D011
200 AND #$7F
210 STA $D011
220 LDA #$FA - Set at start of bottom border
230 STA $D012
BAR1
240 LDX #00 - Here we get data for bar1
250 BACK LDA COLS,X
260 STA 53280
270 JSR DELAY - Do a short delay to hold on that colour for a while
280 INX
290 CPX #12
300 BNE BACK - Do again until all bar1 colours are displayed
BAR2
310 LDX #00 - just the same as BAR1
320 BACK1 LDA COLS1,X
330 STA 53280
340 JSR DELAY
350 INX
360 CPX #12
370 BNE BACK1
BAR3
380 LDX #00 - Just the same
390 BACK2 LDA COLS2,X
400 STA 53280
410 JSR DELAY
420 INX
430 CPX #12
440 BNE BACK2
BAR4
450 LDX #00 - Just the same except this time only 11 colours
460 BACK3 LDA COLS3,X
470 STA 53280
480 JSR DELAY
490 INX
500 CPX #11
510 BNE BACK3
These next part produces a short delay to make the bars smooth (try changing the 2 to 4 or another figure)
520 LDY #02
530 DEL NOP - (NOP is an instruction that means No Operation)
540 DEY
550 BNE DEL
560 LDX #00 - Here we reset the border and background colours to BLACK
570 STX 53280
580 STX 53281
THIS IS BAR1 ROTATE
590 LDY COLS - Here we get the first bit of data into Y Reg
600 LOOP LDA COLS+1,X - Here we load into the Acc the next byte of data
610 STA COLS,X - Then store it at one place back in memory
620 INX - Rotate them all one place down
630 CPX #12 (This is the value of the amount of colours)
640 BNE LOOP
650 TYA - Put first value into accumulator
660 STA COLS,X - Then store it at the end of the new rotated table
BAR2 ROTATE
670 LDX #00 - Just the same
680 LDY COLS1
690 LOOP1 LDA COLS1+1,X
700 STA COLS1,X
710 INX
720 CPX #12
730 BNE LOOP1
740 TYA
750 STA COLS1,X
BAR3 ROTATE
760 LDX #00 - Just the same
770 LDY COLS2
780 LOOP2 LDA COLS2+1,X
790 STA COLS2,X
800 INX
810 CPX #12
820 BNE LOOP2
830 TYA
840 STA COLS2,X
BAR4 ROTATE
850 LDX #00 - The same but only 11 colours to rotate
860 LDY COLS3
870 LDA COLS3+1,X
880 STA COLS3,X
890 INX
900 CPX #11
910 BNE LOOP3
920 TYA
930 STA COLS3,X
940 JMP $EA31 - Jump to ROM
DELAY ROUTINE
950 DELAY LDY #05 - The subroutine that holds the short delay
960 DEL1 NOP
970 DEY
980 BNE DEL1
990 RTS - Return from subroutine
THESE ARE THE COLOURS FOR THE BARS
1000 COLS DFB 9,8,7,1,1,7,8,9,0,0,0,0
1010 COLS1 DFB 2,10,7,1,1,7,10,2,0,0,0,0
1020 COLS2 DFB 6,14,7,1,1,7,14,6,0,0,0,0
1030 COLS3 DFB 5,13,7,1,1,7,13,5,0,0,0
Well i hope you like this effect. I'll be back after my exams to write some more lessons.