Copy Link
Add to Bookmark
Report
QuickBasicNews Issue 1: ARRAY
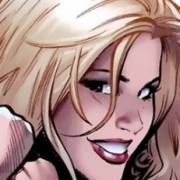
…ÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕª
∫ ARRAYS ∫
»ÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕÕº
For an understanding of arrays as discussed in this section
it is assumed that you have an understanding of how to store
numeric variables and string variables within your program. If
you do not, please review the section at the main menu of
this publication called ( variables ). Then come back to this
section.
Whenever you have a list of related items or a table of items
you should consider putting these items into an Array. Arrays can
either be Single dimensional arrays, or Multidimensional. This months'
column will deal with Single dimensional arrays. Next month we will
go into multidimensional arrays. You might not normally hear an array
called a single dimensional array. Another term used to describe a
single dimensional array is ( simple array ) or just ( array ). From
here on out when we refer to an array we mean a single dimensional array.
This article may seem pretty mundane stuff to the more experienced
programmer. However, keep in mind that these articles are designed
as a progressive type primer to more advanced topics. If you are
an experienced programmer consider this a refresher course.
Even if you don't have much of an idea of what an array is, let me
assure you that you think in arrays all the time. An array is a way
to keep track of related items. We give the array a name. For
example let's give an array the name of February. Now let's assume
that you are a business and you want to keep track of how much
money you take in each day during the month of February. Each of the
days of February can be thought of as an element of February. (1) is an
element of February, (2) is another element and so on. Each element
of an array is identified by the use of a subscript after the variable
name. The Variable name we chose was February. Therefore the first
element ( or the first item ) is February(1), and the second element
is February(2), etc. - down to February(28) .
Now, before we go any further we need to talk about the need to
properly DIMENSION our arrays. A dimension statement simply lets
the computer know how much memory to set aside for our array. If you
do not include a DIMENSION statement to describe the size of your array,
then the array size is limited to a maximum subscript value of 10.
This would mean that your array could have a maximum number of eleven
elements. Where did we get eleven? Well, the first element of
an array is element 0 or arrayname(0), the second element is
arrayname(1), etc. - through arrayname(10). DIMENSION the size of an
array by using the word DIM to determine the size of the array. Be sure
to put the DIM declaratiion before you call on the array. Most often you
will see the DIM declaractions near the beginning of the program before
any executable code.
For example :
DIM February(28) ' sets aside an array named February containing
29 elements. Elements 0 through 28.
In the above example where we want an array to cover each day of
the month of February we could have made our DIM statement
DIM February(27)
And we would have had the 28 elements to cover all 28 days. However,
we might then have to think of the element February(0) as pertaining to
February 1 and element February(1) as relating to February the 2nd..
etc. which could be a bit confusing. It is much easier to think of
the element of February(1) as relating to February the 1st and simply
not use the element February(0).
So far we have been discussing arrays that contain numeric information,
or number values. We can also create string arrays. String arrays
are useful for storing text information ( characters of information )
that contain numbers, and or characters that we do not need to
do math computations with.
An example of a string array might be:
birthdate$(1) = "June 3" (Note that the string of information
should be inside of quotation marks)
And an example of dimensioning a string array might be:
DIM month$(12)
This might be a string array we could use to contain the names
of the twelve months of the year. We could put the name of January
in element one of MONTH$(1) as follows
month$(1) = "January"
If arrays are new to you, it may not be clear to you yet why we
would want to set up an array of month$(1) to be equal to January.
Why wouldn't we just use the name of January whenever we needed it?
Hopefully, this will become clearer as we go along. For now, just
grasp the way arrays are named. The rest will fall into place soon
enough.
Many times you will not know how large to Dimension your array.
An example of this might be a program that keeps track of the
children in a day care center. You would want to add the names
of new children enrolling in the day care center, but you don't want
to have to rewrite the program every time you get a new child. Simply
estimate what the largest size you would need your array to be.
You probably would know the physical size limitations of your
facility might not allow you to have more than 200 children.
Therefore just DIMENSION the array as follows:
DIM names$(200)
As another example - This program you are viewing now was written
in QuickBasic, and each line of the text you are now viewing is an
element of a string array called txt$(). There is no way I knew how
long each article of text would be. However, I estimated that most
articles would probably contain less than 500 lines of text per
article. Therefore I have this DIM statement at the beginning of the
program.
DIM txt$(500)
The important thing to remember, is to DIM your array size large
enough to handle the largest number of elements you may be dealing
with. On the other hand you do not want the array dimensioned to
such a large number that you set aside too much memory for an array
whose elements you won't use anyway. Again, remember if you are
going to use an array with 11 or fewer elements ( elements 0 through
10) then it is not necessary to DIM the array.
If you try to use an element larger than your DIM statement you
will get an error. The message (Subscript out of Range) will be
displayed when you run the program.
Briefly, we will now show you why you might use an array to store
information rather than simply giving each item a different name.
Let's say you wanted to keep track of each child's name entering a day
care center. You could define each child's name this way:
child1$ = "Jennifer Downing age 9 enrolled 8/3/94"
child2$ = "Bobby Turner age 6 enrolled 8/11/94"
child3$ = "Suzie Potter age 8 enrolled 8/13/94"
etc. up to the maximum number of children
Or you could set up an array like this
DIM child$(200)
Now with a simple loop you could input all the children's information
storing each name in an element of the array:
FOR x = 1 to 200
INPUT child$(x)
IF child$(x)= "q" THEN END
Next x
When you run this program it will prompt you for the first element
or child$(1). You simply enter the information and press the enter
key. Then you are prompted for the next name and so on. If you
enter a (q) for a child's name the program will stop. Now each child's
name and information is stored in an array element. If you wanted
to know who was the 16th person enrolled you could do something like
PRINT child$(16)
This is a very simplified description of how to use an array. In
reality the program would include much more information such as
looking to see if the Escape key had been pressed rather
than the (q) key etc. The point here is that you just get a general
grasp as to how you might use an array.
Another thing to remember is that your array names should be
unique. Although not necessary, try to make your names meaningful.
This will help you and others looking at your code. This will
become self evident as you begin to code larger programs.
Do you think the following statements would be allowed within the
same program?
DIM February(28)
DIM February$(28)
Yes, they would be allowed. Although the two array names appear
similar they are different. The key here is the word similar. The
first array will contain numeric information, while the second array
will contain string information.
This column was a basic introduction of arrays. There are a
few other things to learn about arrays which will be touched on next
month. If you understand the simple concepts described above, then
you are well on your way to understanding arrays. Next month we will
continue this discussion of arrays and introduce Multidimensional
arrays.