Chapter 9 - Example programs
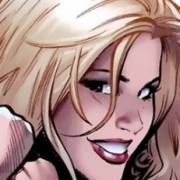
The programs included in this chapter are intended to be illustrations to you in how to write a complete program. The programs are meant to be useful to you either as an example of how to do some operation or as utility programs for your general use.
TIMEDATE - Get Time and Date
This program calls one of the DOS functions to get the current time and date. They are input as variables and can be printed or displayed in any format you desire. Your particular compiler may use a different format because there is no standard in Modula-2. This is one of those areas that will probably deviate from compiler to compiler. If you study your documentation that came with your compiler, you will find many other calls of this type described. This program is meant to be an illustration of how to use this particular call.
TIMEDATE.MOD
MODULE TimeDate;
(* Note - DOSCALL calls interrupt 21(hex) = 33(dec) which is the *)
(* general purpose BIOS interrupt. It is used for many *)
(* operations on the disks, and other peripherals. See *)
(* your DOS manual for details. Some DOS manuals don't *)
(* have any information on this so your next best bet is *)
(* Peter Norton's book "Programmers Guide to the IBM-PC. *)
FROM SYSTEM IMPORT DOSCALL;
FROM InOut IMPORT WriteString, WriteCard, WriteLn;
VAR MonthDay, Year, Month, Day : CARDINAL;
HourMinute, SecondMillisec : CARDINAL;
Hour, Minute, Second, Millisecond : CARDINAL;
Index, Count, Stuff : CARDINAL;
BEGIN
FOR Index := 1 TO 15 DO
DOSCALL(2AH,Year,MonthDay); (* Year is alone in a word *)
Month := MonthDay DIV 256; (* Month is in top half *)
Day := MonthDay MOD 256; (* Day is in bottom half *)
DOSCALL(2CH,HourMinute,SecondMillisec);
Hour := HourMinute DIV 256;
Minute := HourMinute MOD 256;
Second := SecondMillisec DIV 256;
Millisecond := 10*(SecondMillisec MOD 256); (* actually this *)
(* is in hundredths of seconds *)
WriteString("The time is ");
WriteCard(Hour,2);
WriteString(":");
WriteCard(Minute,2);
WriteString(":");
WriteCard(Second,2);
WriteString(":");
WriteCard(Millisecond,3);
WriteString(" ");
WriteCard(Month,2);
WriteString("/");
WriteCard(Day,2);
WriteString("/");
WriteCard(Year,4);
WriteLn;
(* The following loop takes about one second to *)
(* finish its full count on an IBM-PC at normal *)
(* speed. It may vary in time for your system. *)
FOR Count := 1 TO 13300 DO
Stuff := 32000 DIV Count;
END;
END;
END TimeDate.
AREAS - Calculate Areas
This program is intended to be an illustration for you of how to build up a larger program than any other that we have examined up to this point. Notice that the main program is simply one CASE statement that calls all of the other procedures. It would be very simple to include the code from each procedure right in the CASE statement and have no procedure calls, but it would make the program very difficult to understand. The way this example is coded, the code is very easy to understand. After you understand the main program, it is a very simple matter to visit each procedure to see exactly what they do.
Notice how the menu works in this program. It reads one keystroke and responds immediately making it a very simple program to use.
AREAS.MOD
(* This program is actually a very silly program with little or *)
(* no utilitarian value. It is valuable as an illustration of a *)
(* method of implementing a menu for selection purposes. Notice *)
(* when you run it, that the response to your input is immediate *)
(* No "return" is required. *)
MODULE Areas;
FROM InOut IMPORT Read, WriteString, Write, WriteLn;
FROM RealInOut IMPORT WriteReal, ReadReal;
VAR InChar, CapInChar : CHAR;
(* ************************************************** AreaOfSquare *)
PROCEDURE AreaOfSquare;
VAR Length, Area : REAL;
BEGIN
WriteString("Square Enter length of a side ");
ReadReal(Length);
Area := Length * Length;
WriteLn;
WriteString("The area is ");
WriteReal(Area,15);
WriteLn;
END AreaOfSquare;
(* *********************************************** AreaOfRectangle *)
PROCEDURE AreaOfRectangle;
VAR Width, Height, Area : REAL;
BEGIN
WriteString("Rectangle Enter Width ");
ReadReal(Width);
WriteLn;
WriteString("Enter Height ");
ReadReal(Height);
Area := Width * Height;
WriteString(" The area is ");
WriteReal(Area,15);
WriteLn;
END AreaOfRectangle;
(* ************************************************ AreaOfTriangle *)
PROCEDURE AreaOfTriangle;
VAR Base, Height, Area : REAL;
BEGIN
WriteString("Triangle Enter base ");
ReadReal(Base);
WriteLn;
WriteString("Enter height ");
ReadReal(Height);
Area := 0.5 * Base * Height;
WriteString(" The area is ");
WriteReal(Area,15);
WriteLn;
END AreaOfTriangle;
(* ************************************************** AreaOfCIrcle *)
PROCEDURE AreaOfCircle;
VAR Radius, Area : REAL;
BEGIN
WriteString("Circle Enter Radius ");
ReadReal(Radius);
WriteLn;
Area := 3.141592 * Radius * Radius;
WriteString("The area is ");
WriteReal(Area,15);
WriteLn;
END AreaOfCircle;
(* ************************************************** Main Program *)
BEGIN
REPEAT
WriteLn;
WriteString("You only need to input the first letter ");
WriteString("of the selection.");
WriteLn;
WriteString("Select shape; Square Rectangle Triangle ");
WriteString("Circle Quit");
WriteLn;
WriteString("Requested shape is ");
Read(InChar);
CapInChar := CAP(InChar); (* Get capital of letter *)
CASE CapInChar OF
'S' : AreaOfSquare; |
'R' : AreaOfRectangle; |
'T' : AreaOfTriangle; |
'C' : AreaOfCircle; |
'Q' : WriteString("Quit Return to DOS");
WriteLn;
ELSE
Write(InChar);
WriteString(" Invalid Character ");
WriteLn;
END;
UNTIL CapInChar = 'Q';
END Areas.
PC - Printer Control
This is a very useful program that you can use to control your printer. It is specifically set up for an Epson RX-80, but you can modify the control characters to set up your printer to whatever mode you desire. To use the program, you call the program and supply a single letter according to the displayed menu, and the program will send the character or characters to the printer to select the enhanced, compressed, or whatever mode you desire. If your printer is located physically remote from you, you can use this program to send a formfeed to the printer by selecting the F option. If you have some longer control sequences to send, you may want to store the values in a string and use a loop to output the data until you come to an 0C character.
PC.MOD
MODULE PC;
(* The codes in this program are for an EPSON RX-80. You may have *)
(* to adjust the codes for your printer. *)
FROM InOut IMPORT WriteString, WriteLn, Read, Write;
FROM FileSystem IMPORT Lookup, WriteChar, Close, File;
VAR InputChar, Char0, Char1 : CHAR;
PrintFile : File;
BEGIN
WriteString("F Formfeed"); WriteLn;
WriteString("C/N Compressed/Normal"); WriteLn;
WriteString("D/S DoubleStrike/SingleStrike"); WriteLn;
WriteString("E/R Emphasized/Regular"); WriteLn;
WriteLn;
WriteString("Enter Selection --> ");
Read(InputChar);
Write(InputChar);
InputChar := CAP(InputChar);
(* Character input - now output the code *)
CASE InputChar OF
'F' : Char0 := 14C; (* Formfeed *)
Char1 := 0C; |
'C' : Char0 := 17C; (* Compressed mode *)
Char1 := 0C; |
'N' : Char0 := 22C; (* Normal width *)
Char1 := 0C; |
'D' : Char0 := 33C; (* Double Strike - esc-'G' *)
Char1 := 107C; |
'S' : Char0 := 33C; (* Single Strike - esc-'H' *)
Char1 := 110C; |
'E' : Char0 := 33C; (* Emphasized Print - esc-'E' *)
Char1 := 105C; |
'R' : Char0 := 33C; (* Emphasized Off - esc-'@' *)
Char1 := 100C;
ELSE
WriteString("Invalid Character.");
END;
Lookup(PrintFile,"PRN",TRUE); (* Open print file *)
WriteChar(PrintFile,Char0);
IF Char1 > 0C THEN
WriteChar(PrintFile,Char1);
END;
Close(PrintFile);
END PC.
LIST - List Program File
If you ran the batch file named LISTALL as suggested at the beginning of this tutorial to print out all of the source files, you have already used this program. It is the program that will list any ASCII file, adding line numbers, page numbers, and the date and time, on the printer. It is specifically designed to be a program listing utility. The operation is very simple, and you should have no trouble in understanding this program or what it does.
Additional programs will be given at the end of Part III for your information. You will no doubt find additional example programs in various books and periodicals and it would be to your advantage to to spend some time studying them as illustrations of both good and bad practices.
LIST.MOD
MODULE List; (* Program to list Modula-2 source files with page *)
(* numbers and line numbers. *)
FROM FileSystem IMPORT Lookup, Close, File, ReadChar, Response,
WriteChar;
FROM Conversions IMPORT ConvertCardinal;
FROM TimeDate IMPORT GetTime, Time;
IMPORT ASCII;
IMPORT InOut;
TYPE BigString = ARRAY[1..80] OF CHAR;
SmallString = ARRAY[1..25] OF CHAR;
VAR InFile : File; (* The Input File record *)
Printer : File; (* The Printer File record *)
NameOfFile : SmallString; (* Storage for the filename *)
InputLine : BigString; (* The Input line of characters *)
LineNumber : CARDINAL; (* The current line number *)
LinesOnPage : CARDINAL; (* Number of Lines on this page *)
PageNumber : CARDINAL; (* Page Number *)
Index : CARDINAL; (* Used locally in several proc's *)
Year,Day,Month : CARDINAL;
Hour,Minute,Second : CARDINAL;
GoodFile : BOOLEAN;
(* ************************************************ WriteCharString *)
(* Since there is no WriteString procedure in the FileSystem *)
(* module, this procedure does what it would do. It outputs a *)
(* string until it comes to the end of it or until it comes to a *)
(* character 0. *)
PROCEDURE WriteCharString(CharString : ARRAY OF CHAR);
BEGIN
Index := 0;
LOOP
IF Index > HIGH(CharString) THEN EXIT END; (* Max = 80 chars *)
IF CharString[Index] = 0C THEN EXIT END; (* If a 0C is found*)
WriteChar(Printer,CharString[Index]);
INC(Index);
END;
END WriteCharString;
(* ************************************************* WriteLnPrinter *)
(* Since there is no WriteLn procedure in the FileSystem module, *)
(* procedure does its job. *)
PROCEDURE WriteLnPrinter;
CONST CRLF = 12C;
BEGIN
WriteChar(Printer,CRLF);
END WriteLnPrinter;
(* ************************************************* GetFileAndOpen *)
(* This procedure requests the filename, receives it, and opens the *)
(* source file for reading and printing. It loops until a valid *)
(* filename is found. *)
PROCEDURE GetFileAndOpen(VAR GoodFile : BOOLEAN);
BEGIN
InOut.WriteLn;
InOut.WriteString("Name of file to print ---> ");
InOut.ReadString(NameOfFile);
Lookup(InFile,NameOfFile,FALSE);
IF InFile.res = done THEN
GoodFile := TRUE;
Lookup(Printer,"PRN",TRUE); (* open printer as a file *)
ELSE
GoodFile := FALSE;
InOut.WriteString(" File doesn't exist");
InOut.WriteLn;
END;
END GetFileAndOpen;
(* ***************************************************** Initialize *)
(* This procedure initializes some of the counters. *)
PROCEDURE Initialize;
VAR PackedTime : Time;
BEGIN
LineNumber := 1;
LinesOnPage := 0;
PageNumber := 1;
GetTime(PackedTime);
Day := PackedTime.day MOD 32;
Month := PackedTime.day DIV 32;
Month := Month MOD 16;
Year := 1900 + PackedTime.day DIV 512;
Hour := PackedTime.minute DIV 60;
Minute := PackedTime.minute MOD 60;
Second := PackedTime.millisec DIV 1000;
END Initialize;
(* *********************************************** PrintTimeAndDate *)
(* This procedure prints the time and date at the top of every page *)
PROCEDURE PrintTimeAndDate;
VAR OutChars : ARRAY[0..4] OF CHAR;
BEGIN
WriteCharString(" ");
ConvertCardinal(Hour,2,OutChars);
WriteCharString(OutChars);
WriteCharString(":");
ConvertCardinal(Minute,2,OutChars);
WriteCharString(OutChars);
WriteCharString(":");
ConvertCardinal(Second,2,OutChars);
WriteCharString(OutChars);
WriteCharString(" ");
ConvertCardinal(Month,2,OutChars);
WriteCharString(OutChars);
WriteCharString("/");
ConvertCardinal(Day,2,OutChars);
WriteCharString(OutChars);
WriteCharString("/");
ConvertCardinal(Year,4,OutChars);
WriteCharString(OutChars);
END PrintTimeAndDate;
(* *************************************************** OutputHeader *)
(* This procedure prints the filename at the top of each page along *)
(* with the page number. *)
PROCEDURE OutputHeader;
VAR PageOut : ARRAY[1..4] OF CHAR;
BEGIN
WriteCharString(" Filename --> ");
WriteCharString(NameOfFile);
WriteCharString(" ");
PrintTimeAndDate;
WriteCharString(" Page");
ConvertCardinal(PageNumber,4,PageOut);
WriteCharString(PageOut);
WriteLnPrinter;
WriteLnPrinter;
INC(PageNumber);
END OutputHeader;
(* *************************************************** OutputFooter *)
(* This procedure outputs 8 blank lines at the bottom of each page. *)
PROCEDURE OutputFooter;
BEGIN
FOR Index := 1 TO 8 DO
WriteLnPrinter;
END;
END OutputFooter;
(* ******************************************************* GetALine *)
(* This procedure inputs a line from the source file. It quits when*)
(* it finds an end-of-line, an end-of-file, or after it gets 80 *)
(* characters. *)
PROCEDURE GetALine;
VAR LocalChar : CHAR;
BEGIN
FOR Index := 1 TO 80 DO (* clear the input area so that the *)
InputLine[Index] := 0C; (* search for 0C will work. *)
END;
Index := 1;
LOOP
ReadChar(InFile,LocalChar);
IF InFile.eof THEN EXIT END;
InputLine[Index] := LocalChar;
IF LocalChar = ASCII.EOL THEN EXIT END;
INC(Index);
IF Index = 81 THEN EXIT END;
END;
END GetALine;
(* ***************************************************** OutputLine *)
(* Output a line of test with the line number in front of it, after *)
(* checking to see if the page is full. *)
PROCEDURE OutputLine;
VAR Count : CARDINAL;
CardOutArea : ARRAY[1..8] OF CHAR;
BEGIN
INC(LinesOnPage);
IF LinesOnPage > 56 THEN
OutputFooter;
OutputHeader;
LinesOnPage := 1;
END;
ConvertCardinal(LineNumber,6,CardOutArea);
INC(LineNumber);
WriteCharString(CardOutArea);
WriteCharString(" ");
WriteCharString(InputLine);
END OutputLine;
(* *************************************************** SpacePaperUp *)
(* At the end of the listing, space the paper up so that a new page *)
(* is ready for the next listing. *)
PROCEDURE SpacePaperUp;
VAR Count : CARDINAL;
BEGIN
Count := 64 - LinesOnPage;
FOR Index := 1 TO Count DO
WriteLnPrinter;
END;
Close(InFile);
Close(Printer);
END SpacePaperUp;
(* *************************************************** Main Program *)
(* This is nothing more than a big loop. It needs no comment. *)
BEGIN
GetFileAndOpen(GoodFile);
IF GoodFile THEN
Initialize;
OutputHeader;
REPEAT
GetALine;
IF NOT InFile.eof THEN
OutputLine;
END;
UNTIL InFile.eof;
SpacePaperUp;
END;
END List.